Outline of my 2022 SiRAcon 2022 presentation, “Making R work for you
(with automation!)”.
Outline
Use GitHub data to show how the DORA metrics changed over time as I
developed and used rdev.
git log
Idea: use gert::git_log()
tables across all my public
and personal (private) R repositories over time to create an annotated
timeline and visualization of my work, and implementation of the DORA
technical practices.
Import git logs from my repositories:
# gitlogs is now included in siracon2022 for reproducibility, see data-raw/gitlogs and ?gitlogs
gitlogs_tz <- tz(gitlogs$time)
Filter logs by repository, adding cutoff dates when active
development ended for timeline visualization. Drop commits past April 30
to remove partial months.
filtered_gitlogs <- gitlogs |>
# set filter to midnight after last relevant commit, use same timezone as gitlogs
filter(!(repo == "rstudio-training" & time > ymd_h("2020-12-28 0", tz = gitlogs_tz))) |>
filter(!(repo == "software-resilience" & time > ymd_h("2021-02-22 0", tz = gitlogs_tz))) |>
filter(!(repo == "rtraining" & time > ymd_h("2021-10-08 0", tz = gitlogs_tz))) |>
filter(!(repo == "workshop7" & time > ymd_h("2021-12-07 0", tz = gitlogs_tz))) |>
filter(!(repo == "jbplot" & time > ymd_h("2022-02-07 0", tz = gitlogs_tz))) |>
# while this is now redundant (here and elsewhere), keeping it for clarity
filter(time < ymd_h("2022-05-01 0", tz = gitlogs_tz)) |>
# oldest first
mutate(repo = factor(repo, levels = c(
"rstudio-training", "software-resilience", "rtraining", "rdev", "workshop7", "jbplot",
"siracon2022"
)))
Plot monthly commits by repository.
filtered_gitlogs |>
mutate(time = floor_date(time, unit = "month")) |>
group_by(time, repo) |>
summarize(commits = n(), .groups = "drop") |>
ggplot(aes(x = time, y = commits, color = repo)) +
geom_point() +
geom_line() +
labs(title = "Monthly commits by repository") +
labs(x = "", y = "", color = "repository") +
theme_quo()
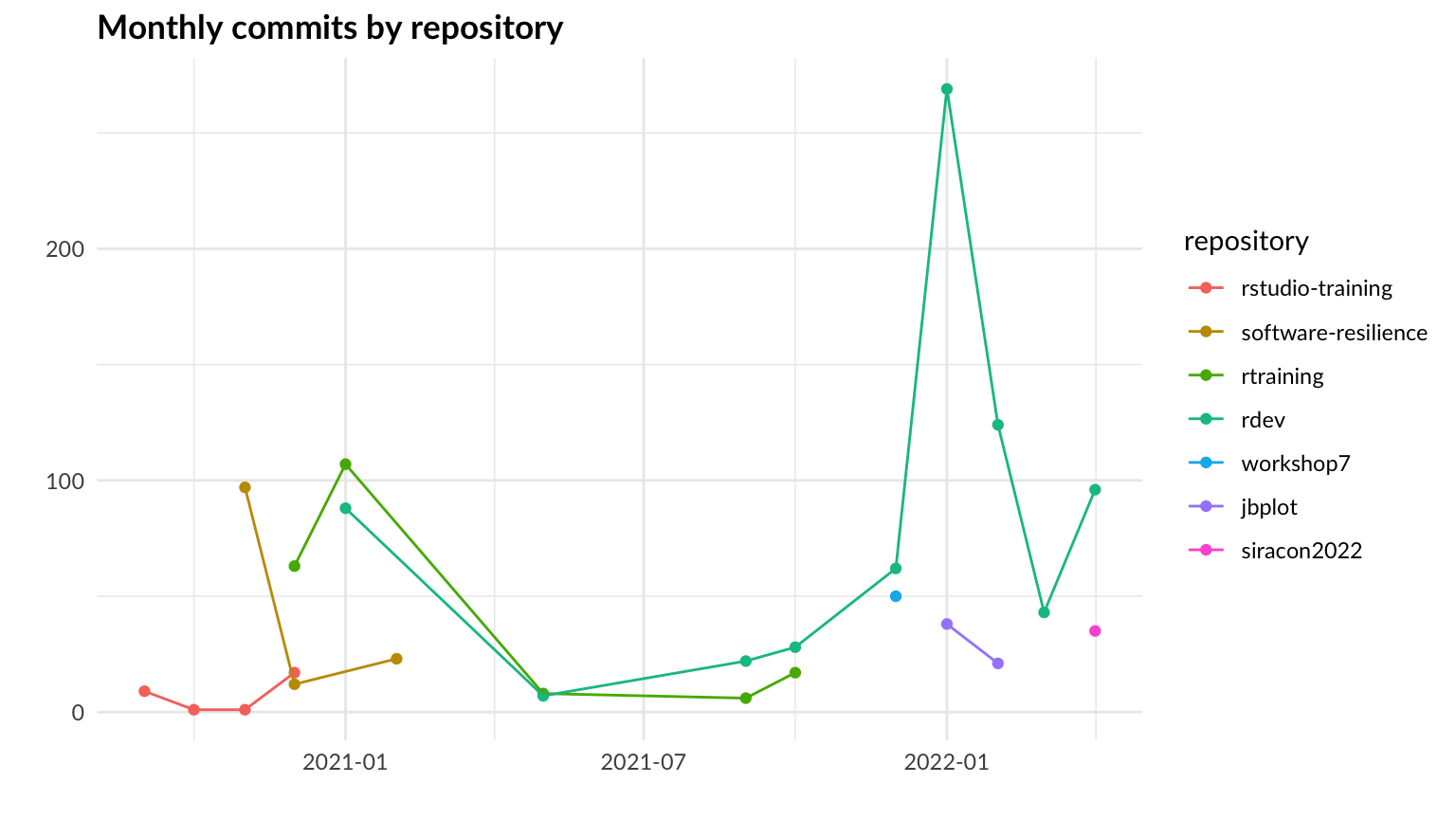
ggsave("rendered/monthly-commits-repo.png", width = 16 * 0.6, height = 9 * 0.6, bg = "white")
High resolution
plot
Timeline
Create a timeline using groups showing the history of the
repositories:
- training: rstudio-training, rtraining
- development: rdev, jbplot
- notebooks: software-resilience, workshop7, siracon2022
repo_timeline <- filtered_gitlogs |>
select(repo, time) |>
mutate(time = floor_date(time, unit = "day")) |>
group_by(repo) |>
summarize(start = min(time), end = max(time)) |>
arrange(start) |>
mutate(
group = case_when(
grepl("training", repo, fixed = TRUE) ~ "training",
repo %in% c("rdev", "jbplot") ~ "development",
TRUE ~ "notebooks"
),
color = hue_pal()(7)[row_number()]
)
repo_timeline
# TODO: gg_vistime doesn't render well when using scale_color_viridis_d()
# issue: https://github.com/shosaco/vistime/issues/30
gg_vistime(repo_timeline, col.event = "repo", title = "R Development Timeline") +
theme_quo()
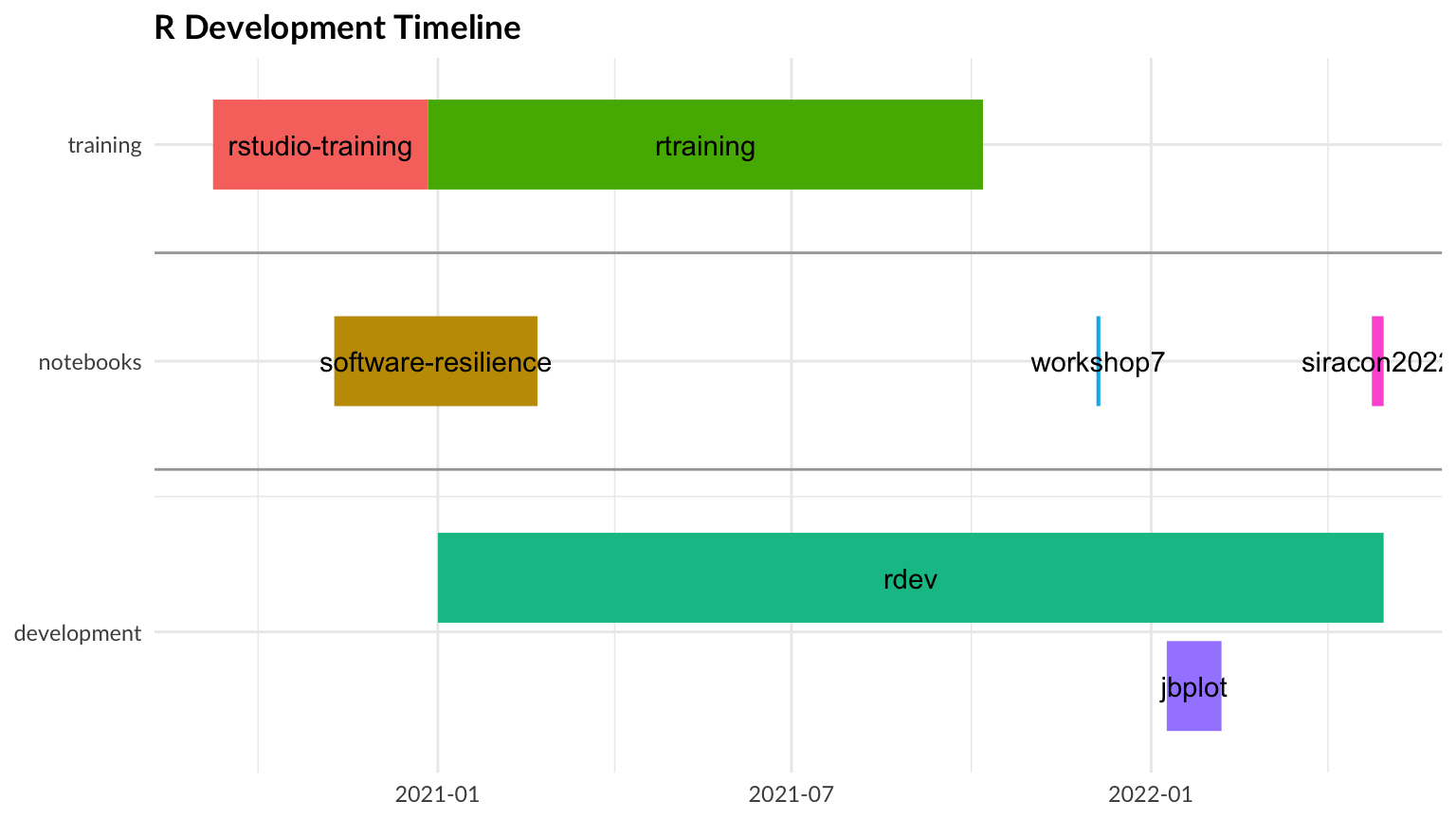
ggsave("rendered/repo-timeline.png", width = 16 * 0.6, height = 9 * 0.6, bg = "white")
High resolution plot
Key events
Plot key events on a timevis()
timeline. Full page version.
key_events <- read_csv("data/key-events.csv", col_types = cols(
id = col_integer(),
start = col_date(format = ""),
end = col_date(format = ""),
content = col_character(),
group = col_integer(),
group_content = col_character(),
intro = col_logical(),
milestone = col_logical()
))
dora_groups <- key_events |>
select(id = group, content = group_content) |>
unique() |>
arrange(id)
render_timevis(key_events, groups = dora_groups, file = "rendered/key-events.html", showZoom = TRUE)
2020-09-08: Starting out, rstudio-training, renv
- Version Control
- Stored project files, notebook (Rmd and html) in private git
repository
- Use renv to store package dependencies in source control
- Trunk-based Development
- Direct commits to master (not recommended)
- Shifting Left on Security
- Start development with
renv::update()
2020-09-11: Published “Working
with R”
2020-09-30: (Aside) First bug discovered, https://github.com/rstudio/renv/issues/547 !
2020-10-06: setup-r
script
- Version Control
- Automate setup of local R development environment
2020-12-02: Adoption of styler and lintr
- Code Maintainability
- Consistent formatting (styler)
- Consistent code (lintr)
- Continuous Testing
- Static code analysis (lintr)
2020-12-27: Migration to rtraining package
- Continuous Integration
- Continuous Testing
- Version Control
2020-12-29: build-site script
- Deployment Automation
- build-site: shell script to publish notebooks using
rmarkdown::render_site()
- MVP for publishing notebooks using GitHub Pages
2020-12-30: First release: rtraining 0.0.1
2020-12-30: GitHub Actions
- Continuous Integration, Continuous Testing
2020-12-30: lint_all()
- Continuous Integration, Continuous Testing
- lint all files locally
- first testthat tests
- roxygen2 documentation
2020-12-30: style_all()
- Continuous Integration, Code Maintainability
- run styler on all files locally
2020-12-31: Switch GitHub Actions to lint_all()
- Continuous Integration, Continuous Testing
- match GitHub and local CI checks
2021-01-01: ci()
, check_renv()
- Continuous Integration, Continuous Testing
- run all CI checks locally
- eliminate toil
- match GitHub and local CI checks
2021-01-01: Migration to rdev package
- Code Maintainability
- Moved most functions to new rdev package
- Consistent tools across projects
2021-01-02: Multi-platform R CMD check
- Continuous Integration, Continuous Testing
- ensure package works on Windows and macOS
2021-01-03: First version of build_analysis_site()
- Deployment Automation
- Automatically build GitHub Pages site with functions, notebooks
- Still a shell script
- Beginning of standard deployment and release pattern:
- bump version
- write code
- update NEWS.md
- “GitHub Release”
- build_site
2021-01-09: Analysis Package Layout
- Code Maintainability
- Consistent package layout across projects
- Supported future automation for creating packages
2021-01-12: Native R version of
build_analysis_site()
2021-01-16: Migrated build_analysis_site()
from
rtraining to rdev
- Code Maintainability
- Cross-platform support
- Moves all automation to R Console
- Deployment Automation
- Automated builds across all projects
2021-09-29: Formal R Analysis Package Layout, Documented release
process
- Code Maintainability
- Consistent package layout across projects
- Supported future automation for creating packages
- Deployment Automation
- Supported future automation for creating releases
2021-12-04: Documented package creation process
- Code Maintainability
- Consistent package layout across projects
- Supported future automation for creating packages
2021-12-23: theme_quo()
: a personalized theme to
visually identify my ggplots.
2022-01-01: Automate package configuration with
use_analysis_package()
- Code Maintainability
- Consistent package layout across projects
2022-01-10: Create package automation (rdev 0.7.0)
create_github_repo()
: Create new GitHub repository
following rdev conventions in the active user’s account and create a
basic package
use_rdev_package()
: Add rdev templates and settings
within the active package. Normally invoked when first setting up a
package.
Added build_rdev_site()
, a wrapper for
pkgdown::build_site()
optimized for rdev workflow that
updates README.md
and performs a clean build using
pkgdown
Added ‘Analysis Notebook’ R markdown template for RStudio (File
> New File > Rmarkdown > From Template)
Migrated ggplot2 themes/styles (theme_quo()
,
viridis_quo()
) to new package,
jabenninghoff/jbplot
Code Maintainability
- Cross-platform support
- Moves all automation to R Console
Deployment Automation
- Automated builds across all projects
2022-01-10: Automate notebook listings in README
library(rdev)
library(fs)
library(dplyr)
library(purrr)
notebooks <- dir_ls("analysis", glob = "*.Rmd") |>
map_dfr(rmd_metadata) |>
mutate(bullet = paste0("- [", title, "](", url, ") (", date, "): ", description)) |>
pull(bullet)
writeLines(notebooks)
2022-01-17: Release automation (rdev 0.8.0)
2022-01-19: More workflow automation
- Added
new_branch()
: Create a new feature branch, and
(optionally) bump the version in DESCRIPTION
2022-01-21 - 2022-02-06: adding test coverage
- Continuous Testing
- Biggest challenge yet
- Significantly improved code quality
- “Unit” testing
- Just test
- Test program flow
- Don’t test other people’s code
- Mock external functions
- Fix bugs by writing a test
- Code coverage, and code coverage metrics
- Test Driven Development
- Tests Give You Confidence (to Refactor)
(Show plot of increasing code coverage from codecov.io)
2022-01-24: write_eval() is a really bad idea:
#' Write and evaluate an expression
#'
#' `write_eval(string)` is a simple wrapper that prints `string` to the console using
#' [`writeLines()`][base::writeLines], then executes the expression using [`parse()`][base::parse]
#' and [`eval()`][base::eval].
#'
#' @param string An expression to be printed to the console and evaluated
#'
#' @return The return value from the evaluated expression
#'
#' @examples
#' write_eval("pi")
#'
#' write_eval("exp(1)")
#' @export
write_eval <- function(string) {
if (!is.character(string)) stop("not a character vector")
if (string == "") stop("nothing to evaluate")
writeLines(string)
eval(parse(text = string))
}
2022-01-30: Manual test script for new package setup
- Continuous Testing
- Manual tests evolve into partially or fully automated tests
2022-02-02: Added local_temppkg()
test helper
function
- Continuous Testing
- Test helpers - testing test helpers helps!
2022-02-06: rdev 1.0.0 !
- Release automation: Stage and create GitHub releases, including
GitHub pages
- Continuous Integration: Local continuous integration checks and
dependency management
- Package Setup: Package setup tasks, typically performed once
2022-02-06 - Today: Continuous Improvement
- Improve CI workflow to catch mistakes
- Spell checks
- Branch protection automation
- Options
- Dependency management
- Product health
Releases
Get releases from GitHub using
siracon2022::gh_releases()
:
# cache results
if (!exists("releases")) {
repos <- c("rtraining", "rdev", "workshop7", "jbplot", "siracon2022")
repos <- setNames(repos, repos)
releases <- map_dfr(repos, gh_releases, "jabenninghoff", .id = "repo") |>
arrange(time)
}
Filter releases past April 30 to remove partial months.
filtered_releases <- releases |>
mutate(time = with_tz(time, tzone = gitlogs_tz)) |>
filter(time < ymd_h("2022-05-01 0", tz = gitlogs_tz))
Plot releases over time: total GitHub releases per period (for all
repositories) to show changes in release frequency. The dotted line
marks the implementation of release automation.
monthly_releases <- filtered_releases |>
mutate(time = floor_date(time, unit = "month")) |>
group_by(time) |>
summarize(releases = n(), .groups = "drop") |>
add_row(time = ymd("2020-11-01"), releases = 0) |>
add_row(time = ymd("2020-10-01"), releases = 0) |>
add_row(time = ymd("2020-09-01"), releases = 0) |>
arrange(time)
monthly_releases |>
ggplot(aes(x = time, y = releases)) +
geom_point() +
geom_line() +
geom_vline(xintercept = ymd_h("2020-12-01 0", tz = gitlogs_tz), linetype = "dotted") +
geom_vline(xintercept = ymd_h("2022-01-01 0", tz = gitlogs_tz), linetype = "dotted") +
coord_cartesian(ylim = c(0, NA)) +
labs(title = "Monthly GitHub releases") +
labs(x = "", y = "") +
theme_quo()
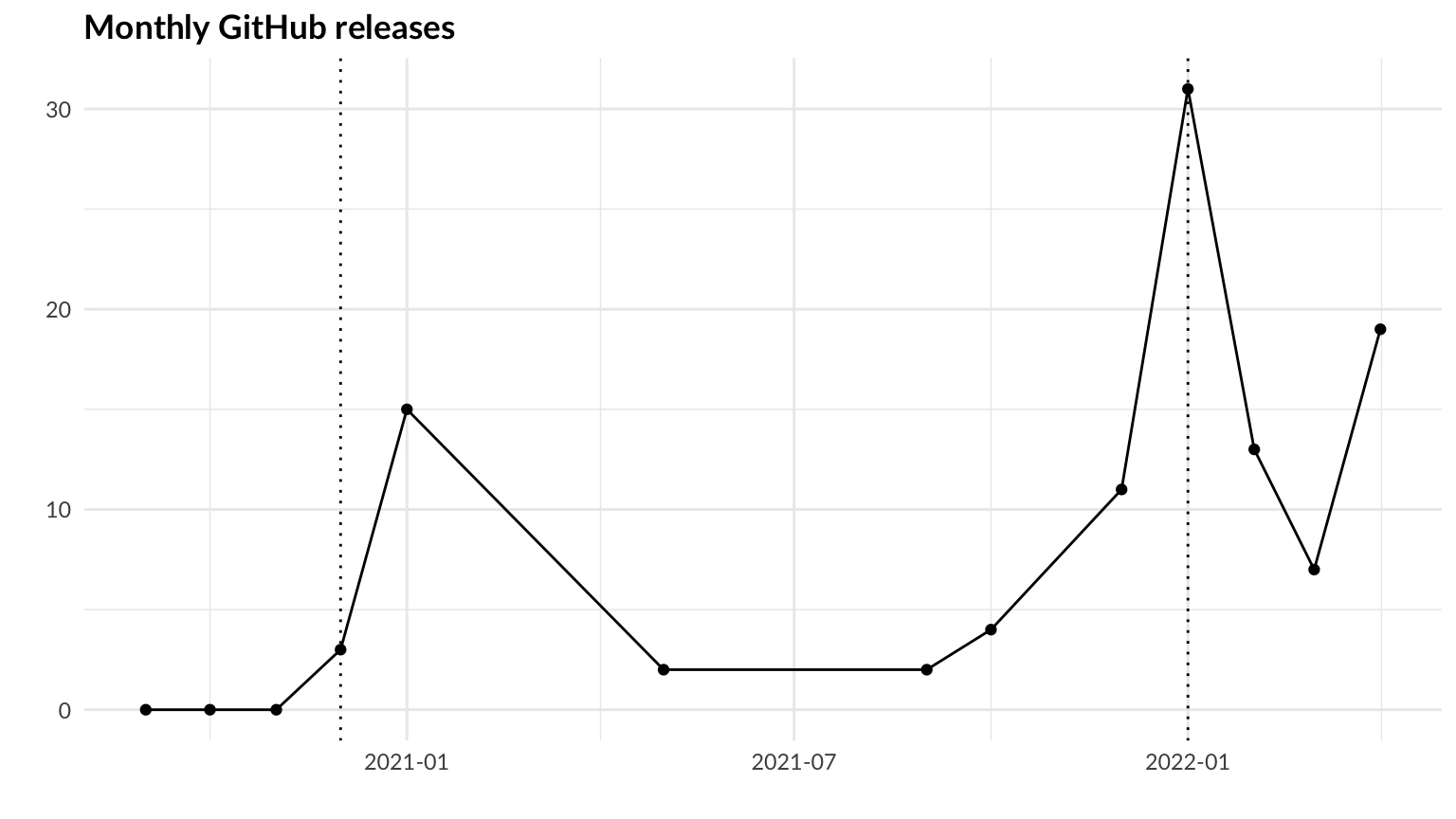
ggsave("rendered/monthly-releases.png", width = 16 * 0.6, height = 9 * 0.6, bg = "white")
High resolution plot
However, the number of releases per month might just represent how
much work is being done, and looks similar to the plot of all commits by
month:
gitlogs |>
filter(time < ymd_h("2022-05-01 0", tz = gitlogs_tz)) |>
mutate(time = floor_date(time, unit = "month")) |>
group_by(time) |>
summarize(commits = n(), .groups = "drop") |>
arrange(time) |>
ggplot(aes(x = time, y = commits)) +
geom_point() +
geom_line() +
geom_vline(xintercept = ymd_h("2020-12-01 0", tz = gitlogs_tz), linetype = "dotted") +
geom_vline(xintercept = ymd_h("2022-01-01 0", tz = gitlogs_tz), linetype = "dotted") +
coord_cartesian(ylim = c(0, NA)) +
labs(title = "Monthly git commits") +
labs(x = "", y = "") +
theme_quo()
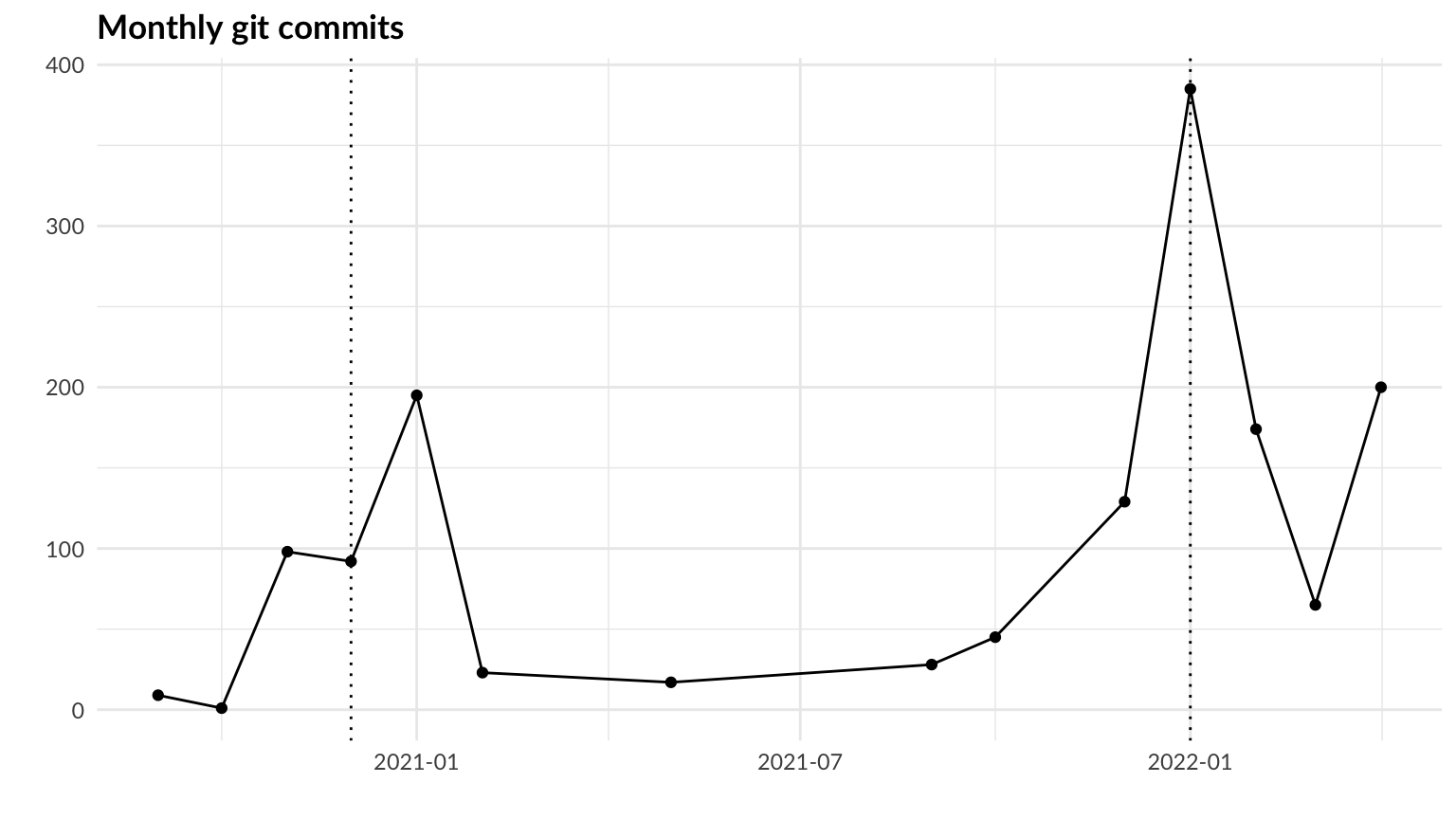
ggsave("rendered/monthly-commits.png", width = 16 * 0.6, height = 9 * 0.6, bg = "white")
High resolution plot
Also plot releases per commit, which will fall between 0 and 1. The
dotted lines mark adoption of GitHub and implementation of release
automation.
gitlogs |>
filter(time < ymd_h("2022-05-01 0", tz = gitlogs_tz)) |>
mutate(time = floor_date(time, unit = "month")) |>
group_by(time) |>
summarize(commits = n()) |>
full_join(monthly_releases, by = "time") |>
replace_na(list(commits = 0, releases = 0)) |>
mutate(rpc = releases / commits) |>
ggplot(aes(x = time, y = rpc)) +
geom_point() +
geom_line() +
geom_vline(xintercept = ymd_h("2020-12-01 0", tz = gitlogs_tz), linetype = "dotted") +
geom_vline(xintercept = ymd_h("2022-01-01 0", tz = gitlogs_tz), linetype = "dotted") +
labs(title = "Monthly GitHub releases per commit") +
labs(x = "", y = "") +
theme_quo()
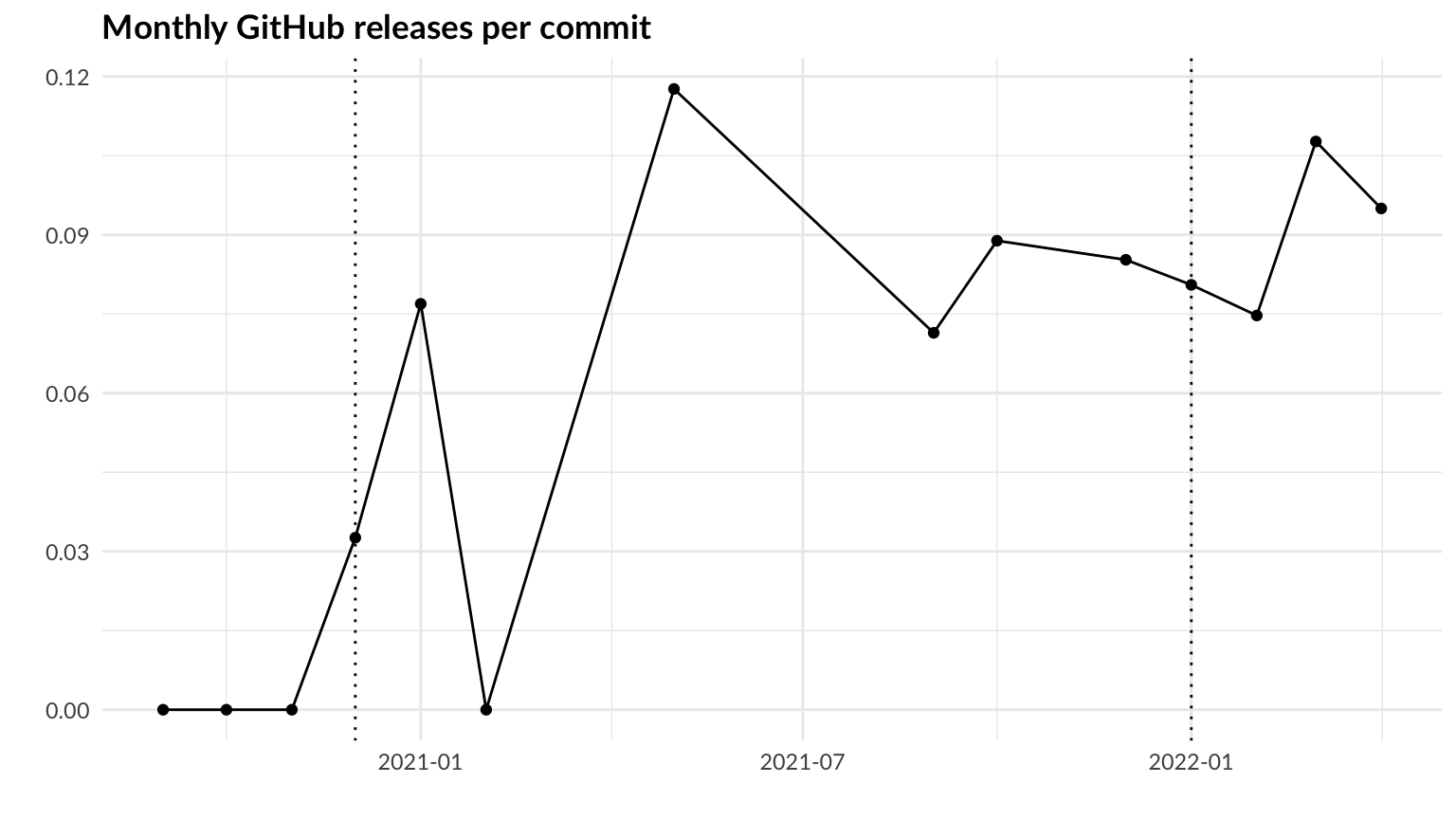
ggsave("rendered/releases-per-commit.png", width = 16 * 0.6, height = 9 * 0.6, bg = "white")
High resolution
plot
Story
Use the timeline and plots to tell the story of continuous
improvement. Each section filters on group 1 and the other focus area.
Integrate themes into story.
- Introduction: background and motivation, use Event group as the talk
overview. Exclude SiRAcon 2020 from future timelines. “R Development
Timeline”.
- Version Control: put everything (except artifacts) into version
control for reproducibility and history.
- Trunk-based Development: linear development avoids code
conflicts.
- Shift Left on Security: maintenance first ensures you get it
done.
- Continuous Integration: build and test on each commit to catch
mistakes early.
- Deployment Automation: automate your development workflow to spend
more time writing.
- Code Maintainability: consistent and clean code is easier to
understand.
- Continuous Testing: (the biggest challenge) formally specifying what
you are building and how it is supposed to work defends against
the dangers of hidden assumptions.
- Results: “Monthly commits by repository”, “Monthly GitHub releases”,
“GitHub releases per commit”. Improvement on technical practices also
means less rework, less deployment pain, less burnout, and greater job
satisfaction.
- Closing: complete key events timeline.
Full rdev package list:
desc
devtools
fs
gert
gh
lintr
markdown
miniUI
pkgdown
purrr
rcmdcheck
remotes
renv
rlang
rmarkdown
styler
tibble
usethis
withr
xml2
yaml
covr
DT
knitr
mockery
spelling
stringi
testthat
Introduction
Background and motivation. Full page
version.
key_events |>
filter(group == 1) |>
render_timevis(groups = filter(dora_groups, id == 1), file = "rendered/intro.html")
Version Control
Put everything (except artifacts) into version control for
reproducibility and history. Full page version.
Use of Homebrew, and
brew bundle
.
Packages:
renv
: dependency management
key_events |>
filter(!intro) |>
filter(milestone | group == 2) |>
render_timevis(groups = filter(dora_groups, id %in% c(1, 2)), "rendered/version-control.html")
Trunk-based Development
Linear development avoids code conflicts. Full page version.
key_events |>
filter(!intro) |>
filter(milestone | group == 3 | id == 44) |>
render_timevis(groups = filter(dora_groups, id %in% c(1, 3)), file = "rendered/trunk-based.html")
Shift Left on Security
Maintenance first ensures you get it done. Full page version.
Reference last year’s talk, recording available in member’s
section.
Packages:
key_events |>
filter(!intro) |>
filter(milestone | group == 4) |>
render_timevis(groups = filter(dora_groups, id %in% c(1, 4)), file = "rendered/shift-left.html")
Continuous Integration
Build and test on each commit to catch mistakes early. Full page version.
Packages:
key_events |>
filter(!intro) |>
filter(milestone | group == 5) |>
render_timevis(groups = filter(dora_groups, id %in% c(1, 5)), file = "rendered/ci.html")
Deployment Automation
Automate your development workflow to spend more time writing. Full page version.
Reducing toil. Forming habits, which become repeated tasks, which
become automation. If it’s automated, it gets done.
Packages:
pkgdown
, rmarkdown
:
build_analysis_site()
gert
, gh
: git, GitHub automation
devtools
key_events |>
filter(!intro) |>
filter(milestone | group == 6 | id == 32) |>
render_timevis(groups = filter(dora_groups, id %in% c(1, 6)), file = "rendered/deployments.html")
Code Maintainability
Consistent and clean code is easier to understand. Full page version.
Functional programming (purrr) vs procedural programming.
Functional programming is harder to learn, but safer.
R dialects: base R is for functions, tidyverse R is for
notebooks.
“Clean” code: code should be written for future humans, including
you!
Packages:
styler
usethis
gh
desc
devtools
purrr
key_events |>
filter(!intro) |>
filter(milestone | group == 7 | id == 32) |>
render_timevis(groups = filter(dora_groups, id %in% c(1, 7)), file = "rendered/code-maint.html")
Continuous Testing
The biggest challenge: formally specifying what you are building and
how it is supposed to work defends against the dangers of
hidden assumptions. Full page
version.
Packages:
lintr
: static code analysis
rcmdcheck
testthat
devtools
covr
mockery
withr
rlang
spelling
Future Testing
Mutation Testing: Wikipedia
R packages:
Papers:
Formal Methods:
key_events |>
filter(!intro) |>
filter(milestone | group == 8 | id == 32) |>
render_timevis(groups = filter(dora_groups, id %in% c(1, 8)), file = "rendered/testing.html")
End of (out)line.
LS0tCnRpdGxlOiBTaVJBY29uIFByZXNlbnRhdGlvbiBPdXRsaW5lCmF1dGhvcjogSm9obiBCZW5uaW5naG9mZgpkYXRlOiAnMjAyMi0wNC0yNCcKZGF0ZS1tb2RpZmllZDogJzIwMjMtMTEtMDgnCmNhdGVnb3JpZXM6IFtdCm9yZGVyOiB+Cm91dHB1dDoKICBodG1sX2RvY3VtZW50OgogICAgdGhlbWU6CiAgICAgIHZlcnNpb246IDUKICAgICAgcHJlc2V0OiBib290c3RyYXAKICAgIGNzczogYXNzZXRzL2V4dHJhLmNzcwogICAgcGFuZG9jX2FyZ3M6IC0tc2hpZnQtaGVhZGluZy1sZXZlbC1ieT0xCiAgICB0b2M6IHllcwogICAgdG9jX2Zsb2F0OgogICAgICBjb2xsYXBzZWQ6IG5vCiAgICAgIHNtb290aF9zY3JvbGw6IG5vCi0tLQoKT3V0bGluZSBvZiBteSAyMDIyIFNpUkFjb24gMjAyMiBwcmVzZW50YXRpb24sICJNYWtpbmcgUiB3b3JrIGZvciB5b3UgKHdpdGggYXV0b21hdGlvbiEpIi4KCiMgUXVlc3Rpb25zL1RPRE8KCi0gWyBdIFF1ZXN0aW9ucy9UT0RPIGxpc3QgaGVyZQoKYGBge3Igc2V0dXAsIG1lc3NhZ2UgPSBGQUxTRSwgd2FybmluZyA9IEZBTFNFfQpsaWJyYXJ5KHNpcmFjb24yMDIyKQpsaWJyYXJ5KHJlYWRyKQpsaWJyYXJ5KHB1cnJyKQpsaWJyYXJ5KGRwbHlyKQpsaWJyYXJ5KHRpZHlyKQpsaWJyYXJ5KGx1YnJpZGF0ZSkKbGlicmFyeShnZ3Bsb3QyKQpsaWJyYXJ5KHNjYWxlcykKbGlicmFyeSh2aXN0aW1lKQpsaWJyYXJ5KGpicGxvdCkKYGBgCgojIEZyYW1ld29yawoKVXNlIHRoZSBbRE9SQSBSZXNlYXJjaCBQcm9ncmFtXShhc3NldHMvZG9yYV9yZXNlYXJjaF9wcm9ncmFtLnBkZikgdG8gZnJhbWUgdGhlIHN0b3J5IG9mIGhvdyBJCmxlYXJuZWQgUiBhbmQgc29mdHdhcmUgZW5naW5lZXJpbmcgYnkgaW1wbGVtZW50aW5nIHRoZSBET1JBIERldk9wcyB0ZWNobmljYWwgcHJhY3RpY2VzOgoKLSBbVmVyc2lvbiBDb250cm9sXShodHRwczovL2Nsb3VkLmdvb2dsZS5jb20vYXJjaGl0ZWN0dXJlL2Rldm9wcy9kZXZvcHMtdGVjaC12ZXJzaW9uLWNvbnRyb2wpCi0gW1RydW5rLWJhc2VkIERldmVsb3BtZW50XShodHRwczovL2Nsb3VkLmdvb2dsZS5jb20vYXJjaGl0ZWN0dXJlL2Rldm9wcy9kZXZvcHMtdGVjaC10cnVuay1iYXNlZC1kZXZlbG9wbWVudCkKLSBbU2hpZnRpbmcgTGVmdCBvbiBTZWN1cml0eV0oaHR0cHM6Ly9jbG91ZC5nb29nbGUuY29tL2FyY2hpdGVjdHVyZS9kZXZvcHMvZGV2b3BzLXRlY2gtc2hpZnRpbmctbGVmdC1vbi1zZWN1cml0eSkKLSBbQ29udGludW91cyBJbnRlZ3JhdGlvbl0oaHR0cHM6Ly9jbG91ZC5nb29nbGUuY29tL2FyY2hpdGVjdHVyZS9kZXZvcHMvZGV2b3BzLXRlY2gtY29udGludW91cy1pbnRlZ3JhdGlvbikKLSBbQ29udGludW91cyBUZXN0aW5nXShodHRwczovL2Nsb3VkLmdvb2dsZS5jb20vYXJjaGl0ZWN0dXJlL2Rldm9wcy9kZXZvcHMtdGVjaC10ZXN0LWF1dG9tYXRpb24pCi0gW0RlcGxveW1lbnQgQXV0b21hdGlvbl0oaHR0cHM6Ly9jbG91ZC5nb29nbGUuY29tL2FyY2hpdGVjdHVyZS9kZXZvcHMvZGV2b3BzLXRlY2gtZGVwbG95bWVudC1hdXRvbWF0aW9uKQotIFtDb2RlIE1haW50YWluYWJpbGl0eV0oaHR0cHM6Ly9jbG91ZC5nb29nbGUuY29tL2FyY2hpdGVjdHVyZS9kZXZvcHMvZGV2b3BzLXRlY2gtY29kZS1tYWludGFpbmFiaWxpdHkpCiAgKGFsc28gY292ZXIgImNsZWFuIiBjb2RlKQoKPGh0dHBzOi8vd3d3LmRldm9wcy1yZXNlYXJjaC5jb20vcmVzZWFyY2guaHRtbD4KCkNyZWF0ZSBhIHRpbWVsaW5lIG9mIG15IGpvdXJuZXkgdXNpbmcgW3Zpc3RpbWVdKGh0dHBzOi8vc2hvc2Fjby5naXRodWIuaW8vdmlzdGltZS8pIG9yClt0aW1ldmlzXShodHRwczovL2RhYXR0YWxpLmNvbS9zaGlueS90aW1ldmlzLWRlbW8vKS4KCiMgT3V0bGluZQoKVXNlIEdpdEh1YiBkYXRhIHRvIHNob3cgaG93IHRoZSBET1JBIG1ldHJpY3MgY2hhbmdlZCBvdmVyIHRpbWUgYXMgSSBkZXZlbG9wZWQgYW5kIHVzZWQKW3JkZXZdKGh0dHBzOi8vamFiZW5uaW5naG9mZi5naXRodWIuaW8vcmRldi8pLgoKIyMgZ2l0IGxvZwoKSWRlYTogdXNlIGBnZXJ0OjpnaXRfbG9nKClgIHRhYmxlcyBhY3Jvc3MgYWxsIG15IHB1YmxpYyBhbmQgcGVyc29uYWwgKHByaXZhdGUpIFIgcmVwb3NpdG9yaWVzIG92ZXIKdGltZSB0byBjcmVhdGUgYW4gYW5ub3RhdGVkIHRpbWVsaW5lIGFuZCB2aXN1YWxpemF0aW9uIG9mIG15IHdvcmssIGFuZCBpbXBsZW1lbnRhdGlvbiBvZiB0aGUgRE9SQQp0ZWNobmljYWwgcHJhY3RpY2VzLgoKSW1wb3J0IGdpdCBsb2dzIGZyb20gbXkgcmVwb3NpdG9yaWVzOgoKYGBge3IgZ2l0bG9nc190en0KIyBnaXRsb2dzIGlzIG5vdyBpbmNsdWRlZCBpbiBzaXJhY29uMjAyMiBmb3IgcmVwcm9kdWNpYmlsaXR5LCBzZWUgZGF0YS1yYXcvZ2l0bG9ncyBhbmQgP2dpdGxvZ3MKCmdpdGxvZ3NfdHogPC0gdHooZ2l0bG9ncyR0aW1lKQpgYGAKCkZpbHRlciBsb2dzIGJ5IHJlcG9zaXRvcnksIGFkZGluZyBjdXRvZmYgZGF0ZXMgd2hlbiBhY3RpdmUgZGV2ZWxvcG1lbnQgZW5kZWQgZm9yIHRpbWVsaW5lCnZpc3VhbGl6YXRpb24uIERyb3AgY29tbWl0cyBwYXN0IEFwcmlsIDMwIHRvIHJlbW92ZSBwYXJ0aWFsIG1vbnRocy4KCmBgYHtyIGZpbHRlcmVkX2dpdGxvZ3N9CmZpbHRlcmVkX2dpdGxvZ3MgPC0gZ2l0bG9ncyB8PgogICMgc2V0IGZpbHRlciB0byBtaWRuaWdodCBhZnRlciBsYXN0IHJlbGV2YW50IGNvbW1pdCwgdXNlIHNhbWUgdGltZXpvbmUgYXMgZ2l0bG9ncwogIGZpbHRlcighKHJlcG8gPT0gInJzdHVkaW8tdHJhaW5pbmciICYgdGltZSA+IHltZF9oKCIyMDIwLTEyLTI4IDAiLCB0eiA9IGdpdGxvZ3NfdHopKSkgfD4KICBmaWx0ZXIoIShyZXBvID09ICJzb2Z0d2FyZS1yZXNpbGllbmNlIiAmIHRpbWUgPiB5bWRfaCgiMjAyMS0wMi0yMiAwIiwgdHogPSBnaXRsb2dzX3R6KSkpIHw+CiAgZmlsdGVyKCEocmVwbyA9PSAicnRyYWluaW5nIiAmIHRpbWUgPiB5bWRfaCgiMjAyMS0xMC0wOCAwIiwgdHogPSBnaXRsb2dzX3R6KSkpIHw+CiAgZmlsdGVyKCEocmVwbyA9PSAid29ya3Nob3A3IiAmIHRpbWUgPiB5bWRfaCgiMjAyMS0xMi0wNyAwIiwgdHogPSBnaXRsb2dzX3R6KSkpIHw+CiAgZmlsdGVyKCEocmVwbyA9PSAiamJwbG90IiAmIHRpbWUgPiB5bWRfaCgiMjAyMi0wMi0wNyAwIiwgdHogPSBnaXRsb2dzX3R6KSkpIHw+CiAgIyB3aGlsZSB0aGlzIGlzIG5vdyByZWR1bmRhbnQgKGhlcmUgYW5kIGVsc2V3aGVyZSksIGtlZXBpbmcgaXQgZm9yIGNsYXJpdHkKICBmaWx0ZXIodGltZSA8IHltZF9oKCIyMDIyLTA1LTAxIDAiLCB0eiA9IGdpdGxvZ3NfdHopKSB8PgogICMgb2xkZXN0IGZpcnN0CiAgbXV0YXRlKHJlcG8gPSBmYWN0b3IocmVwbywgbGV2ZWxzID0gYygKICAgICJyc3R1ZGlvLXRyYWluaW5nIiwgInNvZnR3YXJlLXJlc2lsaWVuY2UiLCAicnRyYWluaW5nIiwgInJkZXYiLCAid29ya3Nob3A3IiwgImpicGxvdCIsCiAgICAic2lyYWNvbjIwMjIiCiAgKSkpCmBgYAoKUGxvdCBtb250aGx5IGNvbW1pdHMgYnkgcmVwb3NpdG9yeS4KCmBgYHtyIG1vbnRobHlfY29tbWl0c19yZXBvfQpmaWx0ZXJlZF9naXRsb2dzIHw+CiAgbXV0YXRlKHRpbWUgPSBmbG9vcl9kYXRlKHRpbWUsIHVuaXQgPSAibW9udGgiKSkgfD4KICBncm91cF9ieSh0aW1lLCByZXBvKSB8PgogIHN1bW1hcml6ZShjb21taXRzID0gbigpLCAuZ3JvdXBzID0gImRyb3AiKSB8PgogIGdncGxvdChhZXMoeCA9IHRpbWUsIHkgPSBjb21taXRzLCBjb2xvciA9IHJlcG8pKSArCiAgZ2VvbV9wb2ludCgpICsKICBnZW9tX2xpbmUoKSArCiAgbGFicyh0aXRsZSA9ICJNb250aGx5IGNvbW1pdHMgYnkgcmVwb3NpdG9yeSIpICsKICBsYWJzKHggPSAiIiwgeSA9ICIiLCBjb2xvciA9ICJyZXBvc2l0b3J5IikgKwogIHRoZW1lX3F1bygpCgpnZ3NhdmUoInJlbmRlcmVkL21vbnRobHktY29tbWl0cy1yZXBvLnBuZyIsIHdpZHRoID0gMTYgKiAwLjYsIGhlaWdodCA9IDkgKiAwLjYsIGJnID0gIndoaXRlIikKYGBgCgpbSGlnaCByZXNvbHV0aW9uIHBsb3RdKHJlbmRlcmVkL21vbnRobHktY29tbWl0cy1yZXBvLnBuZykKCiMjIFRpbWVsaW5lCgpDcmVhdGUgYSB0aW1lbGluZSB1c2luZyBncm91cHMgc2hvd2luZyB0aGUgaGlzdG9yeSBvZiB0aGUgcmVwb3NpdG9yaWVzOgoKLSB0cmFpbmluZzogcnN0dWRpby10cmFpbmluZywgcnRyYWluaW5nCi0gZGV2ZWxvcG1lbnQ6IHJkZXYsIGpicGxvdAotIG5vdGVib29rczogc29mdHdhcmUtcmVzaWxpZW5jZSwgd29ya3Nob3A3LCBzaXJhY29uMjAyMgoKYGBge3IgcmVwb190aW1lbGluZX0KcmVwb190aW1lbGluZSA8LSBmaWx0ZXJlZF9naXRsb2dzIHw+CiAgc2VsZWN0KHJlcG8sIHRpbWUpIHw+CiAgbXV0YXRlKHRpbWUgPSBmbG9vcl9kYXRlKHRpbWUsIHVuaXQgPSAiZGF5IikpIHw+CiAgZ3JvdXBfYnkocmVwbykgfD4KICBzdW1tYXJpemUoc3RhcnQgPSBtaW4odGltZSksIGVuZCA9IG1heCh0aW1lKSkgfD4KICBhcnJhbmdlKHN0YXJ0KSB8PgogIG11dGF0ZSgKICAgIGdyb3VwID0gY2FzZV93aGVuKAogICAgICBncmVwbCgidHJhaW5pbmciLCByZXBvLCBmaXhlZCA9IFRSVUUpIH4gInRyYWluaW5nIiwKICAgICAgcmVwbyAlaW4lIGMoInJkZXYiLCAiamJwbG90IikgfiAiZGV2ZWxvcG1lbnQiLAogICAgICBUUlVFIH4gIm5vdGVib29rcyIKICAgICksCiAgICBjb2xvciA9IGh1ZV9wYWwoKSg3KVtyb3dfbnVtYmVyKCldCiAgKQoKcmVwb190aW1lbGluZQojIFRPRE86IGdnX3Zpc3RpbWUgZG9lc24ndCByZW5kZXIgd2VsbCB3aGVuIHVzaW5nIHNjYWxlX2NvbG9yX3ZpcmlkaXNfZCgpCiMgICBpc3N1ZTogaHR0cHM6Ly9naXRodWIuY29tL3Nob3NhY28vdmlzdGltZS9pc3N1ZXMvMzAKZ2dfdmlzdGltZShyZXBvX3RpbWVsaW5lLCBjb2wuZXZlbnQgPSAicmVwbyIsIHRpdGxlID0gIlIgRGV2ZWxvcG1lbnQgVGltZWxpbmUiKSArCiAgdGhlbWVfcXVvKCkKCmdnc2F2ZSgicmVuZGVyZWQvcmVwby10aW1lbGluZS5wbmciLCB3aWR0aCA9IDE2ICogMC42LCBoZWlnaHQgPSA5ICogMC42LCBiZyA9ICJ3aGl0ZSIpCmBgYAoKW0hpZ2ggcmVzb2x1dGlvbiBwbG90XShyZW5kZXJlZC9yZXBvLXRpbWVsaW5lLnBuZykKCiMjIEtleSBldmVudHMKClBsb3Qga2V5IGV2ZW50cyBvbiBhIGB0aW1ldmlzKClgIHRpbWVsaW5lLiBbRnVsbCBwYWdlIHZlcnNpb25dKHJlbmRlcmVkL2tleS1ldmVudHMuaHRtbCkuCgpgYGB7ciBrZXlfZXZlbnRzLCBmaWcud2lkdGggPSAxMCwgZmlnLmhlaWdodCA9IDE4fQprZXlfZXZlbnRzIDwtIHJlYWRfY3N2KCJkYXRhL2tleS1ldmVudHMuY3N2IiwgY29sX3R5cGVzID0gY29scygKICBpZCA9IGNvbF9pbnRlZ2VyKCksCiAgc3RhcnQgPSBjb2xfZGF0ZShmb3JtYXQgPSAiIiksCiAgZW5kID0gY29sX2RhdGUoZm9ybWF0ID0gIiIpLAogIGNvbnRlbnQgPSBjb2xfY2hhcmFjdGVyKCksCiAgZ3JvdXAgPSBjb2xfaW50ZWdlcigpLAogIGdyb3VwX2NvbnRlbnQgPSBjb2xfY2hhcmFjdGVyKCksCiAgaW50cm8gPSBjb2xfbG9naWNhbCgpLAogIG1pbGVzdG9uZSA9IGNvbF9sb2dpY2FsKCkKKSkKCmRvcmFfZ3JvdXBzIDwtIGtleV9ldmVudHMgfD4KICBzZWxlY3QoaWQgPSBncm91cCwgY29udGVudCA9IGdyb3VwX2NvbnRlbnQpIHw+CiAgdW5pcXVlKCkgfD4KICBhcnJhbmdlKGlkKQoKcmVuZGVyX3RpbWV2aXMoa2V5X2V2ZW50cywgZ3JvdXBzID0gZG9yYV9ncm91cHMsIGZpbGUgPSAicmVuZGVyZWQva2V5LWV2ZW50cy5odG1sIiwgc2hvd1pvb20gPSBUUlVFKQpgYGAKCjIwMjAtMDktMDg6IFN0YXJ0aW5nIG91dCwgcnN0dWRpby10cmFpbmluZywgcmVudgoKLSBWZXJzaW9uIENvbnRyb2wKICAtIFN0b3JlZCBwcm9qZWN0IGZpbGVzLCBub3RlYm9vayAoUm1kIGFuZCBodG1sKSBpbiBwcml2YXRlIGdpdCByZXBvc2l0b3J5CiAgLSBVc2UgcmVudiB0byBzdG9yZSBwYWNrYWdlIGRlcGVuZGVuY2llcyBpbiBzb3VyY2UgY29udHJvbAotIFRydW5rLWJhc2VkIERldmVsb3BtZW50CiAgLSBEaXJlY3QgY29tbWl0cyB0byBtYXN0ZXIgKG5vdCByZWNvbW1lbmRlZCkKLSBTaGlmdGluZyBMZWZ0IG9uIFNlY3VyaXR5CiAgLSBTdGFydCBkZXZlbG9wbWVudCB3aXRoIGByZW52Ojp1cGRhdGUoKWAKCjIwMjAtMDktMTE6IFB1Ymxpc2hlZCBbIldvcmtpbmcgd2l0aCBSIl0oaHR0cHM6Ly93d3cuaW5mb3JtYXRpb24tc2FmZXR5Lm9yZy8yMDIwLzA5LzExL3dvcmtpbmctd2l0aC1yLykKCjIwMjAtMDktMzA6IChBc2lkZSkgRmlyc3QgYnVnIGRpc2NvdmVyZWQsIGh0dHBzOi8vZ2l0aHViLmNvbS9yc3R1ZGlvL3JlbnYvaXNzdWVzLzU0NyAhCgoyMDIwLTEwLTA2OiBgc2V0dXAtcmAgc2NyaXB0CgotIFZlcnNpb24gQ29udHJvbAogIC0gQXV0b21hdGUgc2V0dXAgb2YgbG9jYWwgUiBkZXZlbG9wbWVudCBlbnZpcm9ubWVudAoKMjAyMC0xMi0wMjogQWRvcHRpb24gb2Ygc3R5bGVyIGFuZCBsaW50cgoKLSBDb2RlIE1haW50YWluYWJpbGl0eQogIC0gQ29uc2lzdGVudCBmb3JtYXR0aW5nIChzdHlsZXIpCiAgLSBDb25zaXN0ZW50IGNvZGUgKGxpbnRyKQotIENvbnRpbnVvdXMgVGVzdGluZwogIC0gU3RhdGljIGNvZGUgYW5hbHlzaXMgKGxpbnRyKQoKMjAyMC0xMi0yNzogTWlncmF0aW9uIHRvIHJ0cmFpbmluZyBwYWNrYWdlCgotIENvbnRpbnVvdXMgSW50ZWdyYXRpb24KICAtIEJ1aWxkIFBhY2thZ2UKLSBDb250aW51b3VzIFRlc3RpbmcKICAtIFIgQ01EIGNoZWNrCi0gVmVyc2lvbiBDb250cm9sCiAgLSAuUnByb2ZpbGUKCjIwMjAtMTItMjk6IGJ1aWxkLXNpdGUgc2NyaXB0CgotIERlcGxveW1lbnQgQXV0b21hdGlvbgogIC0gYnVpbGQtc2l0ZTogc2hlbGwgc2NyaXB0IHRvIHB1Ymxpc2ggbm90ZWJvb2tzIHVzaW5nIGBybWFya2Rvd246OnJlbmRlcl9zaXRlKClgCiAgLSBNVlAgZm9yIHB1Ymxpc2hpbmcgbm90ZWJvb2tzIHVzaW5nIEdpdEh1YiBQYWdlcwoKMjAyMC0xMi0zMDogRmlyc3QgcmVsZWFzZTogcnRyYWluaW5nIDAuMC4xCgoyMDIwLTEyLTMwOiBHaXRIdWIgQWN0aW9ucwoKLSBDb250aW51b3VzIEludGVncmF0aW9uLCBDb250aW51b3VzIFRlc3RpbmcKICAtIFItQ01ELWNoZWNrCiAgLSBsaW50cgoKMjAyMC0xMi0zMDogYGxpbnRfYWxsKClgCgotIENvbnRpbnVvdXMgSW50ZWdyYXRpb24sIENvbnRpbnVvdXMgVGVzdGluZwogIC0gbGludCBhbGwgZmlsZXMgbG9jYWxseQogIC0gZmlyc3QgdGVzdHRoYXQgdGVzdHMKICAtIHJveHlnZW4yIGRvY3VtZW50YXRpb24KCjIwMjAtMTItMzA6IGBzdHlsZV9hbGwoKWAKCi0gQ29udGludW91cyBJbnRlZ3JhdGlvbiwgQ29kZSBNYWludGFpbmFiaWxpdHkKICAtIHJ1biBzdHlsZXIgb24gYWxsIGZpbGVzIGxvY2FsbHkKCjIwMjAtMTItMzE6IFN3aXRjaCBHaXRIdWIgQWN0aW9ucyB0byBgbGludF9hbGwoKWAKCi0gQ29udGludW91cyBJbnRlZ3JhdGlvbiwgQ29udGludW91cyBUZXN0aW5nCiAgLSBtYXRjaCBHaXRIdWIgYW5kIGxvY2FsIENJIGNoZWNrcwoKMjAyMS0wMS0wMTogYGNpKClgLCBgY2hlY2tfcmVudigpYAoKLSBDb250aW51b3VzIEludGVncmF0aW9uLCBDb250aW51b3VzIFRlc3RpbmcKICAtIHJ1biBhbGwgQ0kgY2hlY2tzIGxvY2FsbHkKICAtIGVsaW1pbmF0ZSB0b2lsCiAgLSBtYXRjaCBHaXRIdWIgYW5kIGxvY2FsIENJIGNoZWNrcwoKMjAyMS0wMS0wMTogTWlncmF0aW9uIHRvIHJkZXYgcGFja2FnZQoKLSBDb2RlIE1haW50YWluYWJpbGl0eQogIC0gTW92ZWQgbW9zdCBmdW5jdGlvbnMgdG8gbmV3IHJkZXYgcGFja2FnZQogIC0gQ29uc2lzdGVudCB0b29scyBhY3Jvc3MgcHJvamVjdHMKCjIwMjEtMDEtMDI6IE11bHRpLXBsYXRmb3JtIFIgQ01EIGNoZWNrCgotIENvbnRpbnVvdXMgSW50ZWdyYXRpb24sIENvbnRpbnVvdXMgVGVzdGluZwogIC0gZW5zdXJlIHBhY2thZ2Ugd29ya3Mgb24gV2luZG93cyBhbmQgbWFjT1MKCjIwMjEtMDEtMDM6IEZpcnN0IHZlcnNpb24gb2YgYGJ1aWxkX2FuYWx5c2lzX3NpdGUoKWAKCi0gRGVwbG95bWVudCBBdXRvbWF0aW9uCiAgLSBBdXRvbWF0aWNhbGx5IGJ1aWxkIEdpdEh1YiBQYWdlcyBzaXRlIHdpdGggZnVuY3Rpb25zLCBub3RlYm9va3MKICAtIFN0aWxsIGEgc2hlbGwgc2NyaXB0CiAgLSBCZWdpbm5pbmcgb2Ygc3RhbmRhcmQgZGVwbG95bWVudCBhbmQgcmVsZWFzZSBwYXR0ZXJuOgogICAgLSBidW1wIHZlcnNpb24KICAgIC0gd3JpdGUgY29kZQogICAgLSB1cGRhdGUgTkVXUy5tZAogICAgLSAiR2l0SHViIFJlbGVhc2UiCiAgICAtIGJ1aWxkX3NpdGUKCjIwMjEtMDEtMDk6IEFuYWx5c2lzIFBhY2thZ2UgTGF5b3V0CgotIENvZGUgTWFpbnRhaW5hYmlsaXR5CiAgLSBDb25zaXN0ZW50IHBhY2thZ2UgbGF5b3V0IGFjcm9zcyBwcm9qZWN0cwogIC0gU3VwcG9ydGVkIGZ1dHVyZSBhdXRvbWF0aW9uIGZvciBjcmVhdGluZyBwYWNrYWdlcwoKMjAyMS0wMS0xMjogTmF0aXZlIFIgdmVyc2lvbiBvZiBgYnVpbGRfYW5hbHlzaXNfc2l0ZSgpYAoKMjAyMS0wMS0xNjogTWlncmF0ZWQgYGJ1aWxkX2FuYWx5c2lzX3NpdGUoKWAgZnJvbSBydHJhaW5pbmcgdG8gcmRldgoKLSBDb2RlIE1haW50YWluYWJpbGl0eQogIC0gQ3Jvc3MtcGxhdGZvcm0gc3VwcG9ydAogIC0gTW92ZXMgYWxsIGF1dG9tYXRpb24gdG8gUiBDb25zb2xlCi0gRGVwbG95bWVudCBBdXRvbWF0aW9uCiAgLSBBdXRvbWF0ZWQgYnVpbGRzIGFjcm9zcyBhbGwgcHJvamVjdHMKCjIwMjEtMDktMjk6IEZvcm1hbCBSIEFuYWx5c2lzIFBhY2thZ2UgTGF5b3V0LCBEb2N1bWVudGVkIHJlbGVhc2UgcHJvY2VzcwoKLSBDb2RlIE1haW50YWluYWJpbGl0eQogIC0gQ29uc2lzdGVudCBwYWNrYWdlIGxheW91dCBhY3Jvc3MgcHJvamVjdHMKICAtIFN1cHBvcnRlZCBmdXR1cmUgYXV0b21hdGlvbiBmb3IgY3JlYXRpbmcgcGFja2FnZXMKLSBEZXBsb3ltZW50IEF1dG9tYXRpb24KICAtIFN1cHBvcnRlZCBmdXR1cmUgYXV0b21hdGlvbiBmb3IgY3JlYXRpbmcgcmVsZWFzZXMKCjIwMjEtMTItMDQ6IERvY3VtZW50ZWQgcGFja2FnZSBjcmVhdGlvbiBwcm9jZXNzCgotIENvZGUgTWFpbnRhaW5hYmlsaXR5CiAgLSBDb25zaXN0ZW50IHBhY2thZ2UgbGF5b3V0IGFjcm9zcyBwcm9qZWN0cwogIC0gU3VwcG9ydGVkIGZ1dHVyZSBhdXRvbWF0aW9uIGZvciBjcmVhdGluZyBwYWNrYWdlcwoKMjAyMS0xMi0yMzogYHRoZW1lX3F1bygpYDogYSBwZXJzb25hbGl6ZWQgdGhlbWUgdG8gdmlzdWFsbHkgaWRlbnRpZnkgbXkgZ2dwbG90cy4KCjIwMjItMDEtMDE6IEF1dG9tYXRlIHBhY2thZ2UgY29uZmlndXJhdGlvbiB3aXRoIGB1c2VfYW5hbHlzaXNfcGFja2FnZSgpYAoKLSBDb2RlIE1haW50YWluYWJpbGl0eQogIC0gQ29uc2lzdGVudCBwYWNrYWdlIGxheW91dCBhY3Jvc3MgcHJvamVjdHMKCjIwMjItMDEtMTA6IENyZWF0ZSBwYWNrYWdlIGF1dG9tYXRpb24gKHJkZXYgMC43LjApCgotIGBjcmVhdGVfZ2l0aHViX3JlcG8oKWA6IENyZWF0ZSBuZXcgR2l0SHViIHJlcG9zaXRvcnkgZm9sbG93aW5nIHJkZXYgY29udmVudGlvbnMgaW4gdGhlIGFjdGl2ZQogIHVzZXIncyBhY2NvdW50IGFuZCBjcmVhdGUgYSBiYXNpYyBwYWNrYWdlCi0gYHVzZV9yZGV2X3BhY2thZ2UoKWA6IEFkZCByZGV2IHRlbXBsYXRlcyBhbmQgc2V0dGluZ3Mgd2l0aGluIHRoZSBhY3RpdmUgcGFja2FnZS4gTm9ybWFsbHkgaW52b2tlZAogIHdoZW4gZmlyc3Qgc2V0dGluZyB1cCBhIHBhY2thZ2UuCi0gQWRkZWQgYGJ1aWxkX3JkZXZfc2l0ZSgpYCwgYSB3cmFwcGVyIGZvciBgcGtnZG93bjo6YnVpbGRfc2l0ZSgpYCBvcHRpbWl6ZWQgZm9yIHJkZXYgd29ya2Zsb3cgdGhhdAogIHVwZGF0ZXMgYFJFQURNRS5tZGAgYW5kIHBlcmZvcm1zIGEgY2xlYW4gYnVpbGQgdXNpbmcgcGtnZG93bgotIEFkZGVkICdBbmFseXNpcyBOb3RlYm9vaycgUiBtYXJrZG93biB0ZW1wbGF0ZSBmb3IgUlN0dWRpbyAoRmlsZSA+IE5ldyBGaWxlID4gUm1hcmtkb3duID4gRnJvbQogIFRlbXBsYXRlKQotIE1pZ3JhdGVkIGdncGxvdDIgdGhlbWVzL3N0eWxlcyAoYHRoZW1lX3F1bygpYCwgYHZpcmlkaXNfcXVvKClgKSB0byBuZXcgcGFja2FnZSwKICBgamFiZW5uaW5naG9mZi9qYnBsb3RgCgotIENvZGUgTWFpbnRhaW5hYmlsaXR5CiAgLSBDcm9zcy1wbGF0Zm9ybSBzdXBwb3J0CiAgLSBNb3ZlcyBhbGwgYXV0b21hdGlvbiB0byBSIENvbnNvbGUKLSBEZXBsb3ltZW50IEF1dG9tYXRpb24KICAtIEF1dG9tYXRlZCBidWlsZHMgYWNyb3NzIGFsbCBwcm9qZWN0cwoKMjAyMi0wMS0xMDogQXV0b21hdGUgbm90ZWJvb2sgbGlzdGluZ3MgaW4gUkVBRE1FCgotIERlcGxveW1lbnQgQXV0b21hdGlvbgoKYGBgcgpsaWJyYXJ5KHJkZXYpCmxpYnJhcnkoZnMpCmxpYnJhcnkoZHBseXIpCmxpYnJhcnkocHVycnIpCgpub3RlYm9va3MgPC0gZGlyX2xzKCJhbmFseXNpcyIsIGdsb2IgPSAiKi5SbWQiKSB8PgogIG1hcF9kZnIocm1kX21ldGFkYXRhKSB8PgogIG11dGF0ZShidWxsZXQgPSBwYXN0ZTAoIi0gWyIsIHRpdGxlLCAiXSgiLCB1cmwsICIpICgiLCBkYXRlLCAiKTogIiwgZGVzY3JpcHRpb24pKSB8PgogIHB1bGwoYnVsbGV0KQoKd3JpdGVMaW5lcyhub3RlYm9va3MpCmBgYAoKMjAyMi0wMS0xNzogUmVsZWFzZSBhdXRvbWF0aW9uIChyZGV2IDAuOC4wKQoKLSBgc3RhZ2VfcmVsZWFzZSgpYDogT3BlbiBhIEdpdEh1YiBwdWxsIHJlcXVlc3QgZm9yIGEgbmV3IHJlbGVhc2UgZnJvbSBORVdTLm1kCi0gYG1lcmdlX3JlbGVhc2UoKWA6IE1lcmdlIGEgc3RhZ2VkIHB1bGwgcmVxdWVzdCBhbmQgY3JlYXRlIGEgbmV3IEdpdEh1YiByZWxlYXNlCgotIERlcGxveW1lbnQgQXV0b21hdGlvbgoKMjAyMi0wMS0xOTogTW9yZSB3b3JrZmxvdyBhdXRvbWF0aW9uCgotIEFkZGVkIGBuZXdfYnJhbmNoKClgOiBDcmVhdGUgYSBuZXcgZmVhdHVyZSBicmFuY2gsIGFuZCAob3B0aW9uYWxseSkgYnVtcCB0aGUgdmVyc2lvbiBpbgogIERFU0NSSVBUSU9OCgoyMDIyLTAxLTIxIC0gMjAyMi0wMi0wNjogYWRkaW5nIHRlc3QgY292ZXJhZ2UKCi0gQ29udGludW91cyBUZXN0aW5nCiAgLSBCaWdnZXN0IGNoYWxsZW5nZSB5ZXQKICAtIFNpZ25pZmljYW50bHkgaW1wcm92ZWQgY29kZSBxdWFsaXR5CiAgLSAiVW5pdCIgdGVzdGluZwogIC0gSnVzdCB0ZXN0CiAgLSBUZXN0IHByb2dyYW0gZmxvdwogIC0gRG9uJ3QgdGVzdCBvdGhlciBwZW9wbGUncyBjb2RlCiAgLSBNb2NrIGV4dGVybmFsIGZ1bmN0aW9ucwogIC0gRml4IGJ1Z3MgYnkgd3JpdGluZyBhIHRlc3QKICAtIENvZGUgY292ZXJhZ2UsIGFuZCBjb2RlIGNvdmVyYWdlIG1ldHJpY3MKICAtIFRlc3QgRHJpdmVuIERldmVsb3BtZW50CiAgLSBUZXN0cyBHaXZlIFlvdSBDb25maWRlbmNlICh0byBSZWZhY3RvcikKCihTaG93IHBsb3Qgb2YgaW5jcmVhc2luZyBjb2RlIGNvdmVyYWdlIGZyb20gY29kZWNvdi5pbykKCjIwMjItMDEtMjQ6IHdyaXRlX2V2YWwoKSBpcyBhIHJlYWxseSBiYWQgaWRlYToKCmBgYHIKIycgV3JpdGUgYW5kIGV2YWx1YXRlIGFuIGV4cHJlc3Npb24KIycKIycgYHdyaXRlX2V2YWwoc3RyaW5nKWAgaXMgYSBzaW1wbGUgd3JhcHBlciB0aGF0IHByaW50cyBgc3RyaW5nYCB0byB0aGUgY29uc29sZSB1c2luZwojJyAgIFtgd3JpdGVMaW5lcygpYF1bYmFzZTo6d3JpdGVMaW5lc10sIHRoZW4gZXhlY3V0ZXMgdGhlIGV4cHJlc3Npb24gdXNpbmcgW2BwYXJzZSgpYF1bYmFzZTo6cGFyc2VdCiMnICAgYW5kIFtgZXZhbCgpYF1bYmFzZTo6ZXZhbF0uCiMnCiMnIEBwYXJhbSBzdHJpbmcgQW4gZXhwcmVzc2lvbiB0byBiZSBwcmludGVkIHRvIHRoZSBjb25zb2xlIGFuZCBldmFsdWF0ZWQKIycKIycgQHJldHVybiBUaGUgcmV0dXJuIHZhbHVlIGZyb20gdGhlIGV2YWx1YXRlZCBleHByZXNzaW9uCiMnCiMnIEBleGFtcGxlcwojJyB3cml0ZV9ldmFsKCJwaSIpCiMnCiMnIHdyaXRlX2V2YWwoImV4cCgxKSIpCiMnIEBleHBvcnQKd3JpdGVfZXZhbCA8LSBmdW5jdGlvbihzdHJpbmcpIHsKICBpZiAoIWlzLmNoYXJhY3RlcihzdHJpbmcpKSBzdG9wKCJub3QgYSBjaGFyYWN0ZXIgdmVjdG9yIikKICBpZiAoc3RyaW5nID09ICIiKSBzdG9wKCJub3RoaW5nIHRvIGV2YWx1YXRlIikKICB3cml0ZUxpbmVzKHN0cmluZykKICBldmFsKHBhcnNlKHRleHQgPSBzdHJpbmcpKQp9CmBgYAoKMjAyMi0wMS0zMDogTWFudWFsIHRlc3Qgc2NyaXB0IGZvciBuZXcgcGFja2FnZSBzZXR1cAoKLSBDb250aW51b3VzIFRlc3RpbmcKICAtIE1hbnVhbCB0ZXN0cyBldm9sdmUgaW50byBwYXJ0aWFsbHkgb3IgZnVsbHkgYXV0b21hdGVkIHRlc3RzCgoyMDIyLTAyLTAyOiBBZGRlZCBgbG9jYWxfdGVtcHBrZygpYCB0ZXN0IGhlbHBlciBmdW5jdGlvbgoKLSBDb250aW51b3VzIFRlc3RpbmcKICAtIFRlc3QgaGVscGVycyAtIHRlc3RpbmcgdGVzdCBoZWxwZXJzIGhlbHBzIQoKMjAyMi0wMi0wNjogcmRldiAxLjAuMCAhCgotIFJlbGVhc2UgYXV0b21hdGlvbjogU3RhZ2UgYW5kIGNyZWF0ZSBHaXRIdWIgcmVsZWFzZXMsIGluY2x1ZGluZyBHaXRIdWIgcGFnZXMKLSBDb250aW51b3VzIEludGVncmF0aW9uOiBMb2NhbCBjb250aW51b3VzIGludGVncmF0aW9uIGNoZWNrcyBhbmQgZGVwZW5kZW5jeSBtYW5hZ2VtZW50Ci0gUGFja2FnZSBTZXR1cDogUGFja2FnZSBzZXR1cCB0YXNrcywgdHlwaWNhbGx5IHBlcmZvcm1lZCBvbmNlCgoyMDIyLTAyLTA2IC0gVG9kYXk6IENvbnRpbnVvdXMgSW1wcm92ZW1lbnQKCi0gSW1wcm92ZSBDSSB3b3JrZmxvdyB0byBjYXRjaCBtaXN0YWtlcwotIFNwZWxsIGNoZWNrcwotIEJyYW5jaCBwcm90ZWN0aW9uIGF1dG9tYXRpb24KLSBPcHRpb25zCi0gRGVwZW5kZW5jeSBtYW5hZ2VtZW50Ci0gUHJvZHVjdCBoZWFsdGgKCiMjIFJlbGVhc2VzCgpHZXQgcmVsZWFzZXMgZnJvbSBHaXRIdWIgdXNpbmcgYHNpcmFjb24yMDIyOjpnaF9yZWxlYXNlcygpYDoKCmBgYHtyIHJlbGVhc2VzfQojIGNhY2hlIHJlc3VsdHMKaWYgKCFleGlzdHMoInJlbGVhc2VzIikpIHsKICByZXBvcyA8LSBjKCJydHJhaW5pbmciLCAicmRldiIsICJ3b3Jrc2hvcDciLCAiamJwbG90IiwgInNpcmFjb24yMDIyIikKICByZXBvcyA8LSBzZXROYW1lcyhyZXBvcywgcmVwb3MpCiAgcmVsZWFzZXMgPC0gbWFwX2RmcihyZXBvcywgZ2hfcmVsZWFzZXMsICJqYWJlbm5pbmdob2ZmIiwgLmlkID0gInJlcG8iKSB8PgogICAgYXJyYW5nZSh0aW1lKQp9CmBgYAoKRmlsdGVyIHJlbGVhc2VzIHBhc3QgQXByaWwgMzAgdG8gcmVtb3ZlIHBhcnRpYWwgbW9udGhzLgoKYGBge3IgZmlsdGVyZWRfcmVsZWFzZXN9CmZpbHRlcmVkX3JlbGVhc2VzIDwtIHJlbGVhc2VzIHw+CiAgbXV0YXRlKHRpbWUgPSB3aXRoX3R6KHRpbWUsIHR6b25lID0gZ2l0bG9nc190eikpIHw+CiAgZmlsdGVyKHRpbWUgPCB5bWRfaCgiMjAyMi0wNS0wMSAwIiwgdHogPSBnaXRsb2dzX3R6KSkKYGBgCgpQbG90IHJlbGVhc2VzIG92ZXIgdGltZTogdG90YWwgR2l0SHViIHJlbGVhc2VzIHBlciBwZXJpb2QgKGZvciBhbGwgcmVwb3NpdG9yaWVzKSB0byBzaG93IGNoYW5nZXMgaW4KcmVsZWFzZSBmcmVxdWVuY3kuIFRoZSBkb3R0ZWQgbGluZSBtYXJrcyB0aGUgaW1wbGVtZW50YXRpb24gb2YgcmVsZWFzZSBhdXRvbWF0aW9uLgoKYGBge3IgbW9udGhseV9yZWxlYXNlc30KbW9udGhseV9yZWxlYXNlcyA8LSBmaWx0ZXJlZF9yZWxlYXNlcyB8PgogIG11dGF0ZSh0aW1lID0gZmxvb3JfZGF0ZSh0aW1lLCB1bml0ID0gIm1vbnRoIikpIHw+CiAgZ3JvdXBfYnkodGltZSkgfD4KICBzdW1tYXJpemUocmVsZWFzZXMgPSBuKCksIC5ncm91cHMgPSAiZHJvcCIpIHw+CiAgYWRkX3Jvdyh0aW1lID0geW1kKCIyMDIwLTExLTAxIiksIHJlbGVhc2VzID0gMCkgfD4KICBhZGRfcm93KHRpbWUgPSB5bWQoIjIwMjAtMTAtMDEiKSwgcmVsZWFzZXMgPSAwKSB8PgogIGFkZF9yb3codGltZSA9IHltZCgiMjAyMC0wOS0wMSIpLCByZWxlYXNlcyA9IDApIHw+CiAgYXJyYW5nZSh0aW1lKQoKbW9udGhseV9yZWxlYXNlcyB8PgogIGdncGxvdChhZXMoeCA9IHRpbWUsIHkgPSByZWxlYXNlcykpICsKICBnZW9tX3BvaW50KCkgKwogIGdlb21fbGluZSgpICsKICBnZW9tX3ZsaW5lKHhpbnRlcmNlcHQgPSB5bWRfaCgiMjAyMC0xMi0wMSAwIiwgdHogPSBnaXRsb2dzX3R6KSwgbGluZXR5cGUgPSAiZG90dGVkIikgKwogIGdlb21fdmxpbmUoeGludGVyY2VwdCA9IHltZF9oKCIyMDIyLTAxLTAxIDAiLCB0eiA9IGdpdGxvZ3NfdHopLCBsaW5ldHlwZSA9ICJkb3R0ZWQiKSArCiAgY29vcmRfY2FydGVzaWFuKHlsaW0gPSBjKDAsIE5BKSkgKwogIGxhYnModGl0bGUgPSAiTW9udGhseSBHaXRIdWIgcmVsZWFzZXMiKSArCiAgbGFicyh4ID0gIiIsIHkgPSAiIikgKwogIHRoZW1lX3F1bygpCgpnZ3NhdmUoInJlbmRlcmVkL21vbnRobHktcmVsZWFzZXMucG5nIiwgd2lkdGggPSAxNiAqIDAuNiwgaGVpZ2h0ID0gOSAqIDAuNiwgYmcgPSAid2hpdGUiKQpgYGAKCltIaWdoIHJlc29sdXRpb24gcGxvdF0ocmVuZGVyZWQvbW9udGhseS1yZWxlYXNlcy5wbmcpCgpIb3dldmVyLCB0aGUgbnVtYmVyIG9mIHJlbGVhc2VzIHBlciBtb250aCBtaWdodCBqdXN0IHJlcHJlc2VudCBob3cgbXVjaCB3b3JrIGlzIGJlaW5nIGRvbmUsIGFuZApsb29rcyBzaW1pbGFyIHRvIHRoZSBwbG90IG9mIGFsbCBjb21taXRzIGJ5IG1vbnRoOgoKYGBge3IgbW9udGhseV9jb21taXRzfQpnaXRsb2dzIHw+CiAgZmlsdGVyKHRpbWUgPCB5bWRfaCgiMjAyMi0wNS0wMSAwIiwgdHogPSBnaXRsb2dzX3R6KSkgfD4KICBtdXRhdGUodGltZSA9IGZsb29yX2RhdGUodGltZSwgdW5pdCA9ICJtb250aCIpKSB8PgogIGdyb3VwX2J5KHRpbWUpIHw+CiAgc3VtbWFyaXplKGNvbW1pdHMgPSBuKCksIC5ncm91cHMgPSAiZHJvcCIpIHw+CiAgYXJyYW5nZSh0aW1lKSB8PgogIGdncGxvdChhZXMoeCA9IHRpbWUsIHkgPSBjb21taXRzKSkgKwogIGdlb21fcG9pbnQoKSArCiAgZ2VvbV9saW5lKCkgKwogIGdlb21fdmxpbmUoeGludGVyY2VwdCA9IHltZF9oKCIyMDIwLTEyLTAxIDAiLCB0eiA9IGdpdGxvZ3NfdHopLCBsaW5ldHlwZSA9ICJkb3R0ZWQiKSArCiAgZ2VvbV92bGluZSh4aW50ZXJjZXB0ID0geW1kX2goIjIwMjItMDEtMDEgMCIsIHR6ID0gZ2l0bG9nc190eiksIGxpbmV0eXBlID0gImRvdHRlZCIpICsKICBjb29yZF9jYXJ0ZXNpYW4oeWxpbSA9IGMoMCwgTkEpKSArCiAgbGFicyh0aXRsZSA9ICJNb250aGx5IGdpdCBjb21taXRzIikgKwogIGxhYnMoeCA9ICIiLCB5ID0gIiIpICsKICB0aGVtZV9xdW8oKQoKZ2dzYXZlKCJyZW5kZXJlZC9tb250aGx5LWNvbW1pdHMucG5nIiwgd2lkdGggPSAxNiAqIDAuNiwgaGVpZ2h0ID0gOSAqIDAuNiwgYmcgPSAid2hpdGUiKQpgYGAKCltIaWdoIHJlc29sdXRpb24gcGxvdF0ocmVuZGVyZWQvbW9udGhseS1jb21taXRzLnBuZykKCkFsc28gcGxvdCByZWxlYXNlcyBwZXIgY29tbWl0LCB3aGljaCB3aWxsIGZhbGwgYmV0d2VlbiAwIGFuZCAxLiBUaGUgZG90dGVkIGxpbmVzIG1hcmsgYWRvcHRpb24gb2YKR2l0SHViIGFuZCBpbXBsZW1lbnRhdGlvbiBvZiByZWxlYXNlIGF1dG9tYXRpb24uCgpgYGB7ciByZWxlYXNlc19wZXJfY29tbWl0fQpnaXRsb2dzIHw+CiAgZmlsdGVyKHRpbWUgPCB5bWRfaCgiMjAyMi0wNS0wMSAwIiwgdHogPSBnaXRsb2dzX3R6KSkgfD4KICBtdXRhdGUodGltZSA9IGZsb29yX2RhdGUodGltZSwgdW5pdCA9ICJtb250aCIpKSB8PgogIGdyb3VwX2J5KHRpbWUpIHw+CiAgc3VtbWFyaXplKGNvbW1pdHMgPSBuKCkpIHw+CiAgZnVsbF9qb2luKG1vbnRobHlfcmVsZWFzZXMsIGJ5ID0gInRpbWUiKSB8PgogIHJlcGxhY2VfbmEobGlzdChjb21taXRzID0gMCwgcmVsZWFzZXMgPSAwKSkgfD4KICBtdXRhdGUocnBjID0gcmVsZWFzZXMgLyBjb21taXRzKSB8PgogIGdncGxvdChhZXMoeCA9IHRpbWUsIHkgPSBycGMpKSArCiAgZ2VvbV9wb2ludCgpICsKICBnZW9tX2xpbmUoKSArCiAgZ2VvbV92bGluZSh4aW50ZXJjZXB0ID0geW1kX2goIjIwMjAtMTItMDEgMCIsIHR6ID0gZ2l0bG9nc190eiksIGxpbmV0eXBlID0gImRvdHRlZCIpICsKICBnZW9tX3ZsaW5lKHhpbnRlcmNlcHQgPSB5bWRfaCgiMjAyMi0wMS0wMSAwIiwgdHogPSBnaXRsb2dzX3R6KSwgbGluZXR5cGUgPSAiZG90dGVkIikgKwogIGxhYnModGl0bGUgPSAiTW9udGhseSBHaXRIdWIgcmVsZWFzZXMgcGVyIGNvbW1pdCIpICsKICBsYWJzKHggPSAiIiwgeSA9ICIiKSArCiAgdGhlbWVfcXVvKCkKCmdnc2F2ZSgicmVuZGVyZWQvcmVsZWFzZXMtcGVyLWNvbW1pdC5wbmciLCB3aWR0aCA9IDE2ICogMC42LCBoZWlnaHQgPSA5ICogMC42LCBiZyA9ICJ3aGl0ZSIpCmBgYAoKW0hpZ2ggcmVzb2x1dGlvbiBwbG90XShyZW5kZXJlZC9yZWxlYXNlcy1wZXItY29tbWl0LnBuZykKCiMgU3RvcnkKClVzZSB0aGUgdGltZWxpbmUgYW5kIHBsb3RzIHRvIHRlbGwgdGhlIHN0b3J5IG9mIGNvbnRpbnVvdXMgaW1wcm92ZW1lbnQuIEVhY2ggc2VjdGlvbiBmaWx0ZXJzIG9uCmdyb3VwIDEgYW5kIHRoZSBvdGhlciBmb2N1cyBhcmVhLiBJbnRlZ3JhdGUgdGhlbWVzIGludG8gc3RvcnkuCgoxLiBJbnRyb2R1Y3Rpb246IGJhY2tncm91bmQgYW5kIG1vdGl2YXRpb24sIHVzZSBFdmVudCBncm91cCBhcyB0aGUgdGFsayBvdmVydmlldy4gRXhjbHVkZSBTaVJBY29uCiAgIDIwMjAgZnJvbSBmdXR1cmUgdGltZWxpbmVzLiAiUiBEZXZlbG9wbWVudCBUaW1lbGluZSIuCjEuIFZlcnNpb24gQ29udHJvbDogcHV0IGV2ZXJ5dGhpbmcgKGV4Y2VwdCBhcnRpZmFjdHMpIGludG8gdmVyc2lvbiBjb250cm9sIGZvciByZXByb2R1Y2liaWxpdHkgYW5kCiAgIGhpc3RvcnkuCjEuIFRydW5rLWJhc2VkIERldmVsb3BtZW50OiBsaW5lYXIgZGV2ZWxvcG1lbnQgYXZvaWRzIGNvZGUgY29uZmxpY3RzLgoxLiBTaGlmdCBMZWZ0IG9uIFNlY3VyaXR5OiBtYWludGVuYW5jZSBmaXJzdCBlbnN1cmVzIHlvdSBnZXQgaXQgZG9uZS4KMS4gQ29udGludW91cyBJbnRlZ3JhdGlvbjogYnVpbGQgYW5kIHRlc3Qgb24gZWFjaCBjb21taXQgdG8gY2F0Y2ggbWlzdGFrZXMgZWFybHkuCjEuIERlcGxveW1lbnQgQXV0b21hdGlvbjogYXV0b21hdGUgeW91ciBkZXZlbG9wbWVudCB3b3JrZmxvdyB0byBzcGVuZCBtb3JlIHRpbWUgd3JpdGluZy4KMS4gQ29kZSBNYWludGFpbmFiaWxpdHk6IGNvbnNpc3RlbnQgYW5kIGNsZWFuIGNvZGUgaXMgZWFzaWVyIHRvIHVuZGVyc3RhbmQuCjEuIENvbnRpbnVvdXMgVGVzdGluZzogKHRoZSBiaWdnZXN0IGNoYWxsZW5nZSkgZm9ybWFsbHkgc3BlY2lmeWluZyB3aGF0IHlvdSBhcmUgYnVpbGRpbmcgYW5kIGhvdyBpdAogICBpcyAqc3VwcG9zZWQqIHRvIHdvcmsgZGVmZW5kcyBhZ2FpbnN0IHRoZSBkYW5nZXJzIG9mIGhpZGRlbiBhc3N1bXB0aW9ucy4KMS4gUmVzdWx0czogIk1vbnRobHkgY29tbWl0cyBieSByZXBvc2l0b3J5IiwgIk1vbnRobHkgR2l0SHViIHJlbGVhc2VzIiwgIkdpdEh1YiByZWxlYXNlcyBwZXIKICAgY29tbWl0Ii4gSW1wcm92ZW1lbnQgb24gdGVjaG5pY2FsIHByYWN0aWNlcyBhbHNvIG1lYW5zIGxlc3MgcmV3b3JrLCBsZXNzIGRlcGxveW1lbnQgcGFpbiwgbGVzcwogICBidXJub3V0LCBhbmQgZ3JlYXRlciBqb2Igc2F0aXNmYWN0aW9uLgoxLiBDbG9zaW5nOiBjb21wbGV0ZSBrZXkgZXZlbnRzIHRpbWVsaW5lLgoKRnVsbCByZGV2IHBhY2thZ2UgbGlzdDoKCi0gYGRlc2NgCi0gYGRldnRvb2xzYAotIGBmc2AKLSBgZ2VydGAKLSBgZ2hgCi0gYGxpbnRyYAotIGBtYXJrZG93bmAKLSBgbWluaVVJYAotIGBwa2dkb3duYAotIGBwdXJycmAKLSBgcmNtZGNoZWNrYAotIGByZW1vdGVzYAotIGByZW52YAotIGBybGFuZ2AKLSBgcm1hcmtkb3duYAotIGBzdHlsZXJgCi0gYHRpYmJsZWAKLSBgdXNldGhpc2AKLSBgd2l0aHJgCi0gYHhtbDJgCi0gYHlhbWxgCi0gYGNvdnJgCi0gYERUYAotIGBrbml0cmAKLSBgbW9ja2VyeWAKLSBgc3BlbGxpbmdgCi0gYHN0cmluZ2lgCi0gYHRlc3R0aGF0YAoKIyMgSW50cm9kdWN0aW9uCgpCYWNrZ3JvdW5kIGFuZCBtb3RpdmF0aW9uLiBbRnVsbCBwYWdlIHZlcnNpb25dKHJlbmRlcmVkL2ludHJvLmh0bWwpLgoKYGBge3IgaW50cm8sIGZpZy53aWR0aCA9IDguNiwgZmlnLmhlaWdodCA9IDR9CmtleV9ldmVudHMgfD4KICBmaWx0ZXIoZ3JvdXAgPT0gMSkgfD4KICByZW5kZXJfdGltZXZpcyhncm91cHMgPSBmaWx0ZXIoZG9yYV9ncm91cHMsIGlkID09IDEpLCBmaWxlID0gInJlbmRlcmVkL2ludHJvLmh0bWwiKQpgYGAKCiMjIFZlcnNpb24gQ29udHJvbAoKUHV0IGV2ZXJ5dGhpbmcgKGV4Y2VwdCBhcnRpZmFjdHMpIGludG8gdmVyc2lvbiBjb250cm9sIGZvciByZXByb2R1Y2liaWxpdHkgYW5kIGhpc3RvcnkuCltGdWxsIHBhZ2UgdmVyc2lvbl0ocmVuZGVyZWQvdmVyc2lvbi1jb250cm9sLmh0bWwpLgoKKlVzZSBvZiBbSG9tZWJyZXddKGh0dHBzOi8vYnJldy5zaCksIGFuZCBgYnJldyBidW5kbGVgLioKClBhY2thZ2VzOgoKLSBgcmVudmA6IGRlcGVuZGVuY3kgbWFuYWdlbWVudAoKYGBge3IgdmVyc2lvbl9jb250cm9sLCBmaWcud2lkdGggPSA4LjYsIGZpZy5oZWlnaHQgPSAzfQprZXlfZXZlbnRzIHw+CiAgZmlsdGVyKCFpbnRybykgfD4KICBmaWx0ZXIobWlsZXN0b25lIHwgZ3JvdXAgPT0gMikgfD4KICByZW5kZXJfdGltZXZpcyhncm91cHMgPSBmaWx0ZXIoZG9yYV9ncm91cHMsIGlkICVpbiUgYygxLCAyKSksICJyZW5kZXJlZC92ZXJzaW9uLWNvbnRyb2wuaHRtbCIpCmBgYAoKIyMgVHJ1bmstYmFzZWQgRGV2ZWxvcG1lbnQKCkxpbmVhciBkZXZlbG9wbWVudCBhdm9pZHMgY29kZSBjb25mbGljdHMuIFtGdWxsIHBhZ2UgdmVyc2lvbl0ocmVuZGVyZWQvdHJ1bmstYmFzZWQuaHRtbCkuCgpgYGB7ciB0cnVua19iYXNlZCwgZmlnLndpZHRoID0gOC42LCBmaWcuaGVpZ2h0ID0gMi41fQprZXlfZXZlbnRzIHw+CiAgZmlsdGVyKCFpbnRybykgfD4KICBmaWx0ZXIobWlsZXN0b25lIHwgZ3JvdXAgPT0gMyB8IGlkID09IDQ0KSB8PgogIHJlbmRlcl90aW1ldmlzKGdyb3VwcyA9IGZpbHRlcihkb3JhX2dyb3VwcywgaWQgJWluJSBjKDEsIDMpKSwgZmlsZSA9ICJyZW5kZXJlZC90cnVuay1iYXNlZC5odG1sIikKYGBgCgojIyBTaGlmdCBMZWZ0IG9uIFNlY3VyaXR5CgpNYWludGVuYW5jZSBmaXJzdCBlbnN1cmVzIHlvdSBnZXQgaXQgZG9uZS4gW0Z1bGwgcGFnZSB2ZXJzaW9uXShyZW5kZXJlZC9zaGlmdC1sZWZ0Lmh0bWwpLgoKKlJlZmVyZW5jZSBsYXN0IHllYXIncyB0YWxrLCByZWNvcmRpbmcgYXZhaWxhYmxlIGluIG1lbWJlcidzIHNlY3Rpb24uKgoKUGFja2FnZXM6CgotIGByZW52YAoKYGBge3Igc2hpZnRfbGVmdCwgZmlnLndpZHRoID0gOC42LCBmaWcuaGVpZ2h0ID0gMi41fQprZXlfZXZlbnRzIHw+CiAgZmlsdGVyKCFpbnRybykgfD4KICBmaWx0ZXIobWlsZXN0b25lIHwgZ3JvdXAgPT0gNCkgfD4KICByZW5kZXJfdGltZXZpcyhncm91cHMgPSBmaWx0ZXIoZG9yYV9ncm91cHMsIGlkICVpbiUgYygxLCA0KSksIGZpbGUgPSAicmVuZGVyZWQvc2hpZnQtbGVmdC5odG1sIikKYGBgCgojIyBDb250aW51b3VzIEludGVncmF0aW9uCgpCdWlsZCBhbmQgdGVzdCBvbiBlYWNoIGNvbW1pdCB0byBjYXRjaCBtaXN0YWtlcyBlYXJseS4gW0Z1bGwgcGFnZSB2ZXJzaW9uXShyZW5kZXJlZC9jaS5odG1sKS4KClBhY2thZ2VzOgoKLSBgZGV2dG9vbHNgCi0gYHVzZXRoaXNgCi0gW3ItbGliL2FjdGlvbnNdKGh0dHBzOi8vZ2l0aHViLmNvbS9yLWxpYi9hY3Rpb25zKQoKYGBge3IgY2ksIGZpZy53aWR0aCA9IDguNiwgZmlnLmhlaWdodCA9IDMuNX0Ka2V5X2V2ZW50cyB8PgogIGZpbHRlcighaW50cm8pIHw+CiAgZmlsdGVyKG1pbGVzdG9uZSB8IGdyb3VwID09IDUpIHw+CiAgcmVuZGVyX3RpbWV2aXMoZ3JvdXBzID0gZmlsdGVyKGRvcmFfZ3JvdXBzLCBpZCAlaW4lIGMoMSwgNSkpLCBmaWxlID0gInJlbmRlcmVkL2NpLmh0bWwiKQpgYGAKCiMjIERlcGxveW1lbnQgQXV0b21hdGlvbgoKQXV0b21hdGUgeW91ciBkZXZlbG9wbWVudCB3b3JrZmxvdyB0byBzcGVuZCBtb3JlIHRpbWUgd3JpdGluZy4KW0Z1bGwgcGFnZSB2ZXJzaW9uXShyZW5kZXJlZC9kZXBsb3ltZW50cy5odG1sKS4KCipSZWR1Y2luZyB0b2lsLiBGb3JtaW5nIGhhYml0cywgd2hpY2ggYmVjb21lIHJlcGVhdGVkIHRhc2tzLCB3aGljaCBiZWNvbWUgYXV0b21hdGlvbi4gSWYgaXQncwphdXRvbWF0ZWQsIGl0IGdldHMgZG9uZS4qCgpQYWNrYWdlczoKCi0gYHBrZ2Rvd25gLCBgcm1hcmtkb3duYDogYGJ1aWxkX2FuYWx5c2lzX3NpdGUoKWAKLSBgZ2VydGAsIGBnaGA6IGdpdCwgR2l0SHViIGF1dG9tYXRpb24KLSBgZGV2dG9vbHNgCgpgYGB7ciBkZXBsb3ltZW50cywgZmlnLndpZHRoID0gOC42LCBmaWcuaGVpZ2h0ID0gNC41fQprZXlfZXZlbnRzIHw+CiAgZmlsdGVyKCFpbnRybykgfD4KICBmaWx0ZXIobWlsZXN0b25lIHwgZ3JvdXAgPT0gNiB8IGlkID09IDMyKSB8PgogIHJlbmRlcl90aW1ldmlzKGdyb3VwcyA9IGZpbHRlcihkb3JhX2dyb3VwcywgaWQgJWluJSBjKDEsIDYpKSwgZmlsZSA9ICJyZW5kZXJlZC9kZXBsb3ltZW50cy5odG1sIikKYGBgCgojIyBDb2RlIE1haW50YWluYWJpbGl0eQoKQ29uc2lzdGVudCBhbmQgY2xlYW4gY29kZSBpcyBlYXNpZXIgdG8gdW5kZXJzdGFuZC4gW0Z1bGwgcGFnZSB2ZXJzaW9uXShyZW5kZXJlZC9jb2RlLW1haW50Lmh0bWwpLgoKKkZ1bmN0aW9uYWwgcHJvZ3JhbW1pbmcgKHB1cnJyKSB2cyBwcm9jZWR1cmFsIHByb2dyYW1taW5nLiBGdW5jdGlvbmFsIHByb2dyYW1taW5nIGlzIGhhcmRlciB0bwpsZWFybiwgYnV0IHNhZmVyLioKCipSIGRpYWxlY3RzOiBiYXNlIFIgaXMgZm9yIGZ1bmN0aW9ucywgdGlkeXZlcnNlIFIgaXMgZm9yIG5vdGVib29rcy4qCgoqIkNsZWFuIiBjb2RlOiBjb2RlIHNob3VsZCBiZSB3cml0dGVuIGZvciBmdXR1cmUgaHVtYW5zLCBpbmNsdWRpbmcgeW91ISoKClBhY2thZ2VzOgoKLSBgc3R5bGVyYAotIGB1c2V0aGlzYAotIGBnaGAKLSBgZGVzY2AKLSBgZGV2dG9vbHNgCi0gYHB1cnJyYAoKYGBge3IgY29kZV9tYWludCwgZmlnLndpZHRoID0gOC42LCBmaWcuaGVpZ2h0ID0gNX0Ka2V5X2V2ZW50cyB8PgogIGZpbHRlcighaW50cm8pIHw+CiAgZmlsdGVyKG1pbGVzdG9uZSB8IGdyb3VwID09IDcgfCBpZCA9PSAzMikgfD4KICByZW5kZXJfdGltZXZpcyhncm91cHMgPSBmaWx0ZXIoZG9yYV9ncm91cHMsIGlkICVpbiUgYygxLCA3KSksIGZpbGUgPSAicmVuZGVyZWQvY29kZS1tYWludC5odG1sIikKYGBgCgojIyBDb250aW51b3VzIFRlc3RpbmcKClRoZSBiaWdnZXN0IGNoYWxsZW5nZTogZm9ybWFsbHkgc3BlY2lmeWluZyB3aGF0IHlvdSBhcmUgYnVpbGRpbmcgYW5kIGhvdyBpdCBpcyAqc3VwcG9zZWQqIHRvIHdvcmsKZGVmZW5kcyBhZ2FpbnN0IHRoZSBkYW5nZXJzIG9mIGhpZGRlbiBhc3N1bXB0aW9ucy4gW0Z1bGwgcGFnZSB2ZXJzaW9uXShyZW5kZXJlZC90ZXN0aW5nLmh0bWwpLgoKUGFja2FnZXM6CgotIGBsaW50cmA6IHN0YXRpYyBjb2RlIGFuYWx5c2lzCi0gYHJjbWRjaGVja2AKLSBgdGVzdHRoYXRgCi0gYGRldnRvb2xzYAotIGBjb3ZyYAotIGBtb2NrZXJ5YAotIGB3aXRocmAKLSBgcmxhbmdgCi0gYHNwZWxsaW5nYAoKIyMjIEZ1dHVyZSBUZXN0aW5nCgpNdXRhdGlvbiBUZXN0aW5nOiBbV2lraXBlZGlhXShodHRwczovL2VuLndpa2lwZWRpYS5vcmcvd2lraS9NdXRhdGlvbl90ZXN0aW5nKQoKUiBwYWNrYWdlczoKCi0gW211dGFudF0oaHR0cHM6Ly9naXRodWIuY29tL3Nja290dC9tdXRhbnQpCi0gW2F1dG90ZXN0XShodHRwczovL2dpdGh1Yi5jb20vcm9wZW5zY2ktcmV2aWV3LXRvb2xzL2F1dG90ZXN0LykKClBhcGVyczoKCi0gW0RvZXMgbXV0YXRpb24gdGVzdGluZyBpbXByb3ZlIHRlc3RpbmcgcHJhY3RpY2VzP10oaHR0cHM6Ly9ob21lcy5jcy53YXNoaW5ndG9uLmVkdS9+cmp1c3QvcHVibC9tdXRhdGlvbl90ZXN0aW5nX3ByYWN0aWNlc19pY3NlXzIwMjEucGRmKQotIFtQcmFjdGljYWwgTXV0YXRpb24gVGVzdGluZyBhdCBTY2FsZV0oaHR0cHM6Ly9ob21lcy5jcy53YXNoaW5ndG9uLmVkdS9+cmp1c3QvcHVibC9wcmFjdGljYWxfbXV0YXRpb25fdGVzdGluZ190cl8yMDIxLnBkZikKCkZvcm1hbCBNZXRob2RzOgoKLSBbUGxhbm5pbmcgd2l0aCBmbGFyZV0oaHR0cHM6Ly9pbmNyZW1lbnQuY29tL3BsYW5uaW5nL2Zvcm1hbC1zcGVjaWZpY2F0aW9ucy1hbmQtcGxhbm5pbmcvKQotIFtIaWxsZWwgV2F5bmVdKGh0dHBzOi8vaGlsbGVsd2F5bmUuY29tKQotIFtMZWFybiBUTEErXShodHRwczovL2xlYXJudGxhLmNvbS8pCi0gW0FsbG95IERvY3VtZW50YXRpb25dKGh0dHBzOi8vYWxsb3kucmVhZHRoZWRvY3MuaW8vZW4vbGF0ZXN0LykKCmBgYHtyIHRlc3RpbmcsIGZpZy53aWR0aCA9IDguNiwgZmlnLmhlaWdodCA9IDMuNX0Ka2V5X2V2ZW50cyB8PgogIGZpbHRlcighaW50cm8pIHw+CiAgZmlsdGVyKG1pbGVzdG9uZSB8IGdyb3VwID09IDggfCBpZCA9PSAzMikgfD4KICByZW5kZXJfdGltZXZpcyhncm91cHMgPSBmaWx0ZXIoZG9yYV9ncm91cHMsIGlkICVpbiUgYygxLCA4KSksIGZpbGUgPSAicmVuZGVyZWQvdGVzdGluZy5odG1sIikKYGBgCgpFbmQgb2YgKG91dClsaW5lLgo=